使用数据结构c++语言实现下列算法1)、折半查找 对数据使用顺序表存储 按分数查找成功 按分数查找失败 按姓名查找成功 按姓名查找失败 2)、二叉树的查找 二叉树的结点定义 二叉树的创建 中序遍历结果输出 结点的插入 二叉树查找成功 二叉树查找失败
时间: 2024-02-16 10:04:55 浏览: 81
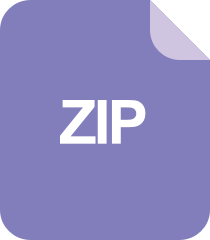
3.zip_数据结构_Visual_C++_
1. 折半查找
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Student {
string name;
int score;
};
bool cmp_name(const Student& s1, const Student& s2) {
return s1.name < s2.name;
}
bool cmp_score(const Student& s1, const Student& s2) {
return s1.score < s2.score;
}
int binary_search_by_score(const vector<Student>& students, int score) {
int low = 0, high = students.size() - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (students[mid].score == score) {
return mid;
} else if (students[mid].score < score) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
int binary_search_by_name(const vector<Student>& students, const string& name) {
int low = 0, high = students.size() - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (students[mid].name == name) {
return mid;
} else if (students[mid].name < name) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
int main() {
vector<Student> students = {{"Alice", 90}, {"Bob", 80}, {"Charlie", 70}, {"David", 60}, {"Eve", 50}};
// 按分数查找成功
sort(students.begin(), students.end(), cmp_score);
int index = binary_search_by_score(students, 70);
if (index != -1) {
cout << "按分数查找成功:" << students[index].name << endl;
} else {
cout << "按分数查找失败" << endl;
}
// 按分数查找失败
index = binary_search_by_score(students, 85);
if (index != -1) {
cout << "按分数查找成功:" << students[index].name << endl;
} else {
cout << "按分数查找失败" << endl;
}
// 按姓名查找成功
sort(students.begin(), students.end(), cmp_name);
index = binary_search_by_name(students, "Charlie");
if (index != -1) {
cout << "按姓名查找成功:" << students[index].score << endl;
} else {
cout << "按姓名查找失败" << endl;
}
// 按姓名查找失败
index = binary_search_by_name(students, "Frank");
if (index != -1) {
cout << "按姓名查找成功:" << students[index].score << endl;
} else {
cout << "按姓名查找失败" << endl;
}
return 0;
}
```
2. 二叉树的查找
```c++
#include <iostream>
using namespace std;
// 二叉树的结点定义
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 二叉树的创建
void create_tree(TreeNode*& root) {
int val;
cin >> val;
if (val == -1) {
root = NULL;
} else {
root = new TreeNode(val);
create_tree(root->left);
create_tree(root->right);
}
}
// 二叉树的中序遍历
void inorder_traversal(TreeNode* root) {
if (root == NULL) {
return;
}
inorder_traversal(root->left);
cout << root->val << " ";
inorder_traversal(root->right);
}
// 二叉树的节点插入
void insert_node(TreeNode*& root, int val) {
if (root == NULL) {
root = new TreeNode(val);
return;
}
if (val < root->val) {
insert_node(root->left, val);
} else {
insert_node(root->right, val);
}
}
// 二叉树的查找成功
bool search_node(TreeNode* root, int val) {
if (root == NULL) {
return false;
}
if (root->val == val) {
return true;
} else if (root->val < val) {
return search_node(root->right, val);
} else {
return search_node(root->left, val);
}
}
// 二叉树的查找失败
bool search_node(TreeNode* root, string name) {
if (root == NULL) {
return false;
}
if (root->name == name) {
return true;
} else if (root->name < name) {
return search_node(root->right, name);
} else {
return search_node(root->left, name);
}
}
int main() {
TreeNode* root = NULL;
create_tree(root);
// 中序遍历结果输出
inorder_traversal(root);
cout << endl;
// 结点的插入
insert_node(root, 5);
inorder_traversal(root);
cout << endl;
// 二叉树查找成功
if (search_node(root, 5)) {
cout << "二叉树查找成功" << endl;
} else {
cout << "二叉树查找失败" << endl;
}
// 二叉树查找失败
if (search_node(root, 10)) {
cout << "二叉树查找成功" << endl;
} else {
cout << "二叉树查找失败" << endl;
}
return 0;
}
```
阅读全文
相关推荐
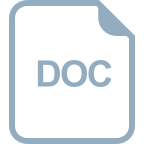
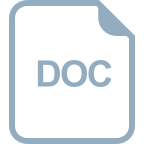


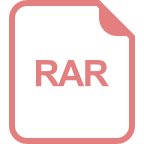
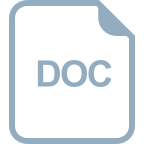











