python中实现以下任务:(a) Load the image 'lena.jpg', convert it to grayscale. (b) Perform sharpening spatial filtering to enhance the image with different parameters (at least 3 kinds). (Tips: skimage.filters.laplace)
时间: 2023-05-19 07:05:08 浏览: 116
可以使用以下代码实现:
```python
import skimage.io as io
import skimage.color as color
import skimage.filters as filters
# Load the image and convert it to grayscale
img = io.imread('lena.jpg')
gray_img = color.rgb2gray(img)
# Perform sharpening spatial filtering with different parameters
sharpened_img1 = gray_img - filters.laplace(gray_img, ksize=3)
sharpened_img2 = gray_img - filters.laplace(gray_img, ksize=5)
sharpened_img3 = gray_img - filters.laplace(gray_img, ksize=7)
```
其中,`ksize`参数表示Laplace算子的大小,越大的值会导致更强的锐化效果。
相关问题
编程实现:读入一幅图像lena.bmp,利用prewitt算子对图像进行锐化,并在图幅中显示
在MATLAB中,你可以使用`imread`函数来读取BMP图像文件,然后使用`prewitt`算子进行边缘检测,最后用`imagesc`或`imshow`函数来显示结果。以下是实现这个过程的步骤:
```matlab
% 1. 读取图像
lena = imread('lena.bmp');
% 2. 检查图像是否为双通道(RGB),如果是,转换为灰度图像
if size(lena, 3) == 3
lena = rgb2gray(lena);
end
% 3. 应用Prewitt算子进行边缘检测
sobelX = [-1 0 1; -1 0 1; -1 0 1]; % Prewitt算子矩阵
lena_edges = imfilter(lena, sobelX, 'conv'); % x方向滤波
lena_edges = abs(lena_edges); % 取绝对值以突出边缘
% 4. 对y方向也应用Prewitt算子,通常两个方向结合起来效果更好
sobelY = [-1 -1 -1; 0 0 0; 1 1 1];
lena_edges = sqrt(double(imfilter(lena_edges, sobelY.' * sobelX, 'conv')^2)); % y方向后平方再开方
% 5. 显示结果
figure;
subplot(1,2,1), imshow(lena, 'InitialMagnification', 'fit');
title('Original Lena Image'), axis off;
subplot(1,2,2), imshow(lena_edges, 'InitialMagnification', 'fit');
title('Edge Detection (Prewitt)'), axis off;
```
这段代码首先读取并显示原图像,然后计算边缘,最后在同一个窗口内分别显示原始图像和处理后的边缘检测结果。
Write a python script to open the “lena.png” file using OpenCV. • Display the opened image in a new window named “Display Lena” • Save the image to a new file named “lena_resaved.png”,其中lena.png存放在D盘data_test文件夹下
下面是一个使用OpenCV打开并显示图像,并将其保存为新文件的Python脚本:
```python
import cv2
# 图像文件路径
image_path = 'D:/data_test/lena.png'
# 使用OpenCV打开图像
image = cv2.imread(image_path)
# 显示图像
cv2.imshow('Display Lena', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 保存图像到新文件
save_path = 'D:/data_test/lena_resaved.png'
cv2.imwrite(save_path, image)
```
在这个脚本中,首先指定了要打开的图像文件的路径 `image_path`,然后使用 `cv2.imread()` 函数读取图像并存储在 `image` 变量中。然后使用 `cv2.imshow()` 显示图像,窗口的名称为 "Display Lena"。通过 `cv2.waitKey(0)` 等待用户按下任意键来关闭显示窗口。最后,使用 `cv2.imwrite()` 函数将图像保存为新文件,保存路径为 `save_path`。
请确保将图像文件 "lena.png" 放在指定的路径 "D:/data_test/" 下,并运行以上代码来打开、显示和保存图像。
阅读全文
相关推荐
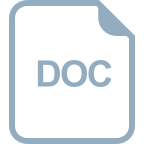
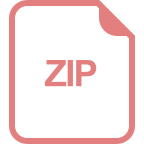
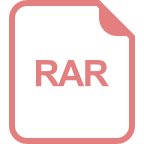
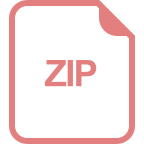
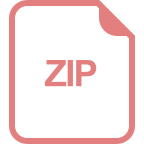
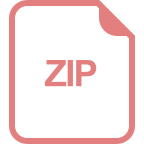
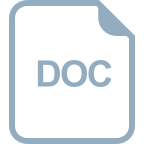






