c++字符串只保留前6位
时间: 2024-02-13 19:04:40 浏览: 137
在C++中,可以使用`std::string`类自带的`substr()`函数来截取子字符串。以下是一个简单的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str;
cout << "Enter a string: ";
getline(cin, str);
string new_str = str.substr(0, 6); // 截取前6个字符
cout << "Resultant string: " << new_str;
return 0;
}
```
该程序从标准输入中读取一个字符串,然后使用`substr()`函数截取该字符串的前6个字符。最后,程序输出结果字符串。注意,`substr()`函数的第一个参数是子字符串的起始位置,从0开始;第二个参数是子字符串的长度。
相关问题
c++输入一个字符串只保留多的字母
首先,我们需要明确一下题目中的“多的字母”是指什么。
如果“多的字母”是指在输入的字符串中出现次数最多的字母,那我们就需要统计每个字母的出现次数,找出出现次数最多的字母,并将其保留下来。
具体做法如下:
1. 创建一个字典,用来存储每个字母的出现次数。
2. 遍历输入的字符串,对于每个字符进行如下操作:
- 如果该字符已经在字典中存在,则将其对应的次数加1;
- 如果该字符不在字典中,那么将其添加到字典中,并将其对应的次数设为1。
3. 找出字典中出现次数最多的字符及其对应的次数。
4. 遍历字符串,将只保留出现次数最多的字符的其他字符删除,形成新的字符串。
5. 输出新的字符串。
下面是实现上述算法的Python代码:
```
def keep_most_frequent_char(input_str):
char_count = {}
for char in input_str:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
max_count = max(char_count.values())
most_frequent_char = ''
for char, count in char_count.items():
if count == max_count:
most_frequent_char = char
break
new_str = ''.join([char for char in input_str if char == most_frequent_char])
return new_str
input_str = input("请输入一个字符串:")
new_str = keep_most_frequent_char(input_str)
print("只保留多的字母后的字符串为:", new_str)
```
希望以上回答能够满足你的需求,如有疑问,请随时追问。
c++字符串存储两位小数
在 C++ 中,如果你想要存储两位小数的字符串,你可以直接使用 `std::string` 类型来保存,因为 C++ 的字符串可以存储任意字符序列,包括数字和点号。例如:
```cpp
#include <iostream>
#include <string>
int main() {
std::string decimalString = "12.34";
// 或者
double decimalValue = 12.34;
std::ostringstream oss;
oss << std::fixed << std::setprecision(2) << decimalValue; // 设置精度到两位
std::string formattedDecimal = oss.str();
std::cout << "String representation of a two-decimal number: " << formattedDecimal << "\n";
return 0;
}
```
在这个例子中,`std::fixed` 用于固定小数点后的位数,`std::setprecision(2)` 设置了小数点后保留两位。然后将 `decimalValue` 转换为字符串并保存在 `formattedDecimal` 中。
阅读全文
相关推荐
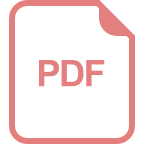
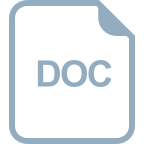
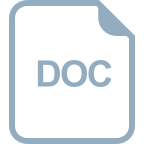








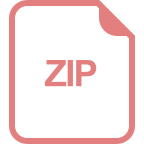