用select()监测GPIO是否发生中断
时间: 2024-01-10 15:05:10 浏览: 126
在 Linux 中,GPIO 可以通过 sysfs 接口来进行访问和控制。如果要使用 `select()` 监测 GPIO 中断,需要将 GPIO 的中断事件文件(通常是 `/sys/class/gpio/gpioX/value`)打开,并将其添加到 `select()` 监测的文件描述符集合中。当 GPIO 中断触发时,对应的中断事件文件将变为可读状态,`select()` 函数就会返回,并且可以通过读取文件来获取中断事件的信息。
以下是一个示例代码片段,用于使用 `select()` 监测 GPIO 中断:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/select.h>
#define GPIO_PIN 18
int main(void) {
char gpio_path[64];
int gpio_fd;
fd_set read_fds;
int ret;
// 打开 GPIO 中断事件文件
snprintf(gpio_path, sizeof(gpio_path), "/sys/class/gpio/gpio%d/value", GPIO_PIN);
gpio_fd = open(gpio_path, O_RDONLY);
if (gpio_fd < 0) {
perror("open");
exit(1);
}
// 初始化文件描述符集合
FD_ZERO(&read_fds);
FD_SET(gpio_fd, &read_fds);
// 循环等待 GPIO 中断
while (1) {
ret = select(gpio_fd + 1, &read_fds, NULL, NULL, NULL);
if (ret < 0) {
perror("select");
exit(1);
} else if (ret == 0) {
// 超时
continue;
} else if (FD_ISSET(gpio_fd, &read_fds)) {
// GPIO 中断触发,读取中断事件
char buf[8];
int len;
lseek(gpio_fd, 0, SEEK_SET);
len = read(gpio_fd, buf, sizeof(buf));
if (len < 0) {
perror("read");
exit(1);
}
// 处理中断事件
printf("GPIO interrupt occurred!\n");
}
}
return 0;
}
```
注意,上述代码仅适用于使用 sysfs 接口控制 GPIO 的情况。如果使用其他方式(如 mmap())访问 GPIO,需要根据具体情况进行修改。
阅读全文
相关推荐
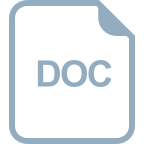
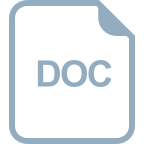
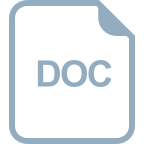

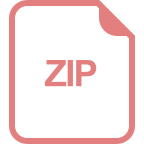
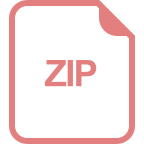
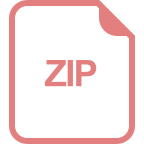
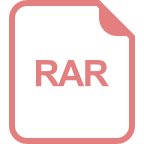
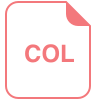
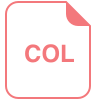
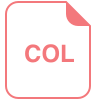
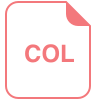
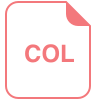

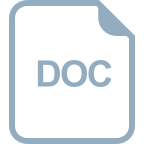
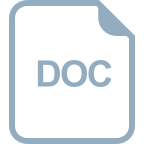
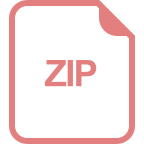