用python实现航迹起始
时间: 2024-11-18 11:14:45 浏览: 3
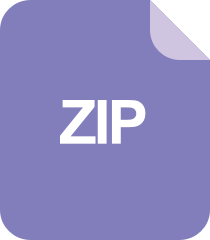
使用matlab开发的M/N逻辑方法,实现航迹起始

在Python中,实现航迹起点通常涉及到数据处理和地理位置的操作。首先,你需要获取航迹点的数据,这可以来自于GPS记录、传感器读取或者其他定位系统。例如,如果你有经纬度坐标列表,你可以使用geopandas库来进行操作。
下面是一个简单的示例,假设你有一个包含时间戳和经纬度的字典列表:
```python
import geopandas as gpd
from shapely.geometry import Point
# 假设你的航迹数据是一个字典列表,每个字典包含'timestamp'(时间戳)和'coordinates'(经纬度)
data = [
{'timestamp': '2022-01-01T00:00:00', 'coordinates': (51.5074, -0.1278)},
# ... 其他时间点和坐标
]
# 将数据转换成GeoDataFrame
points = [{'geometry': Point(xy), 'time': timestamp} for xy, timestamp in data]
gdf = gpd.GeoDataFrame(points)
# 设置时间作为索引以便排序
gdf.set_index('time', inplace=True)
# 航迹起点通常是第一个位置
start_point = gdf.iloc[0]
print(start_point)
```
在这个例子中,`start_point`就是航迹的初始位置。
阅读全文
相关推荐
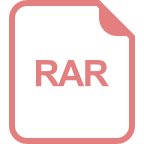
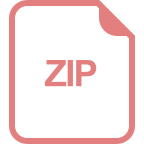
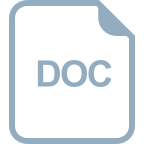


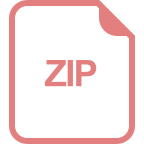
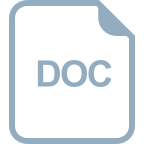
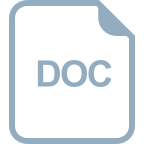
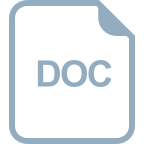



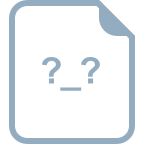
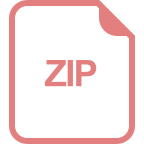
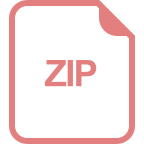