用C++编写一个简单程序,定义一个点类 Point,并定义成员函数 double Distance(const \\& Point) 求两点间的距离,要求不能用std: cont 的形式
时间: 2024-12-06 12:24:32 浏览: 24
在C++中,我们可以手动创建一个简单的点类`Point`,不依赖于`std::vector`或其他容器。首先,我们定义一个`Point`类,包含两个属性表示x坐标和y坐标。然后,我们将创建一个名为`Distance`的纯虚函数,这个函数接受一个`const Point&`参数,用于计算两点之间的欧氏距离。
```cpp
#include <iostream>
using namespace std;
class Point {
public:
// 定义点的坐标
double x;
double y;
// 构造函数
Point(double x = 0, double y = 0) : x(x), y(y) {}
// 纯虚函数,用于计算两点间距离
virtual double Distance(const Point &other) const = 0;
};
// 实现Distance函数的具体版本
class Point2D : public Point {
private:
static const double PI = 3.14159265358979323846;
public:
// 继承Point并实现Distance函数
double Distance(const Point &other) const override {
double dx = this->x - other.x;
double dy = this->y - other.y;
return sqrt(dx * dx + dy * dy);
}
};
int main() {
// 创建两个点
Point2D p1(0, 0);
Point2D p2(3, 4);
// 计算并打印两点间的距离
cout << "Distance between point1 (0, 0) and point2 (3, 4): " << p1.Distance(p2) << endl;
return 0;
}
```
在这个例子中,我们创建了一个`Point2D`类作为`Point`的派生类,并实现了`Distance`函数。在`main`函数中,我们实例化了这两个点并调用了它们之间的距离计算。
阅读全文
相关推荐
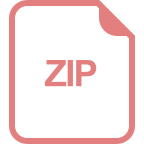
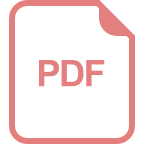
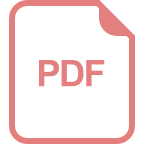















