注释每一行代码import tensorflow as tf mnist = tf.keras.datasets.mnist #加载mnist数据集 (train_images,train_labels),(test_images,test_labels) = mnist.load_data() train_images, test_images = train_images/255.0, test_images/255.0 #归一化到0-1 print("Train image shape:",train_images.shape,"Train label shape:",train_labels.shape) #打印图像大小和标签数 print("Test image shape:",test_images.shape,"Test label shape:",test_labels.shape) print("image data:",train_images[1]) #具体看一幅image的数据 print("label data:",train_labels[1]) import matplotlib.pyplot as plt def plot_image(image): plt.imshow(image.reshape(28,28),cmap='binary') plt.show() plot_image(train_images[1]) #搭建模型 model = tf.keras.models.Sequential([ tf.keras.layers.Flatten(input_shape=[28, 28]), tf.keras.layers.Dense(128, activation='relu'), tf.keras.layers.Dropout(0.2), tf.keras.layers.Dense(10, activation='softmax') ]) #编译 model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) #训练模型 model.fit(train_images,train_labels, epochs=5, batch_size=64) model.evaluate(test_images,test_labels)
时间: 2024-02-13 13:01:32 浏览: 25
以下是对代码的注释:
```python
import tensorflow as tf # 导入TensorFlow库
mnist = tf.keras.datasets.mnist # 加载MNIST数据集
# 加载训练集和测试集,train_images和test_images存储图像数据,train_labels和test_labels存储标签数据
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
train_images, test_images = train_images/255.0, test_images/255.0 # 对图像数据进行归一化处理
print("Train image shape:", train_images.shape, "Train label shape:", train_labels.shape) # 打印训练集图像数据的大小和标签数
print("Test image shape:", test_images.shape, "Test label shape:", test_labels.shape) # 打印测试集图像数据的大小和标签数
print("image data:", train_images[1]) # 打印训练集中第二张图像的数据
print("label data:", train_labels[1]) # 打印训练集中第二张图像的标签
import matplotlib.pyplot as plt # 导入matplotlib库
# 定义函数plot_image,用于显示图像
def plot_image(image):
plt.imshow(image.reshape(28, 28), cmap='binary') # 将图像转换为28*28的矩阵并显示
plt.show()
plot_image(train_images[1]) # 显示训练集中第二张图像
# 搭建神经网络模型,包括输入层、隐藏层和输出层
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=[28, 28]), # 将28*28的图像数据展开成一维数组
tf.keras.layers.Dense(128, activation='relu'), # 添加一个包含128个神经元的隐藏层
tf.keras.layers.Dropout(0.2), # 添加Dropout层,避免过拟合
tf.keras.layers.Dense(10, activation='softmax') # 添加一个包含10个神经元的输出层,用于分类
])
# 编译神经网络模型,选择优化器、损失函数和评估指标
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# 训练神经网络模型,传入训练集数据和标签,设置训练次数和每次训练的批次大小
model.fit(train_images, train_labels, epochs=5, batch_size=64)
# 评估神经网络模型的性能,传入测试集数据和标签
model.evaluate(test_images, test_labels)
```
相关推荐
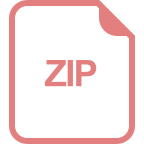
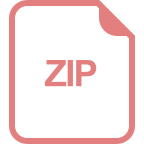
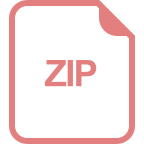














