用c语言实现用栈完成从键盘输入一个算术表达式,完成表达式求值,表达式中数字可以为一位数,表达式中的符号包括加、减、乘、除、小括号;若输入为左括号,在已入栈运算符中优先级最高,在未入栈运算符中最低,相当于新的栈底,若输入加号,在已入栈运算符中仅高于左括号。请输出代码和实验结果
时间: 2024-02-06 17:13:04 浏览: 19
好的,下面是使用 C 语言实现该算法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 20
typedef struct {
int top;
char data[MAX_SIZE];
} Stack;
void push(Stack *s, char c) {
if (s->top == MAX_SIZE - 1) {
printf("Stack overflow\n");
exit(1);
}
s->data[++s->top] = c;
}
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow\n");
exit(1);
}
return s->data[s->top--];
}
char peek(Stack *s) {
if (s->top == -1) {
printf("Stack underflow\n");
exit(1);
}
return s->data[s->top];
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '(' || c == ')';
}
int get_priority(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else if (c == '(') {
return 3;
} else {
return 0;
}
}
int calculate(int a, int b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
default: return 0;
}
}
int evaluate(char *expr) {
Stack num_stack, op_stack;
num_stack.top = -1;
op_stack.top = -1;
int i = 0;
while (expr[i] != '\0') {
if (expr[i] == ' ') {
i++;
continue;
}
if (!is_operator(expr[i])) {
int num = expr[i] - '0';
push(&num_stack, num);
} else {
if (expr[i] == '(' || (op_stack.top == -1 || peek(&op_stack) == '(')) {
push(&op_stack, expr[i]);
} else if (expr[i] == ')') {
while (peek(&op_stack) != '(') {
char op = pop(&op_stack);
int b = pop(&num_stack);
int a = pop(&num_stack);
int result = calculate(a, b, op);
push(&num_stack, result);
}
pop(&op_stack);
} else {
while (op_stack.top != -1 && peek(&op_stack) != '(' && get_priority(peek(&op_stack)) >= get_priority(expr[i])) {
char op = pop(&op_stack);
int b = pop(&num_stack);
int a = pop(&num_stack);
int result = calculate(a, b, op);
push(&num_stack, result);
}
push(&op_stack, expr[i]);
}
}
i++;
}
while (op_stack.top != -1) {
char op = pop(&op_stack);
int b = pop(&num_stack);
int a = pop(&num_stack);
int result = calculate(a, b, op);
push(&num_stack, result);
}
return pop(&num_stack);
}
int main() {
char expr[MAX_SIZE];
printf("Enter an arithmetic expression: ");
fgets(expr, MAX_SIZE, stdin);
int len = strlen(expr);
if (expr[len - 1] == '\n') {
expr[len - 1] = '\0';
}
int result = evaluate(expr);
printf("Result: %d\n", result);
return 0;
}
```
该代码中使用了两个栈:一个用于存储数字,一个用于存储运算符。其中 `push`、`pop`、`peek` 和 `is_empty` 函数分别用于栈的基本操作。`is_operator` 函数用于判断一个字符是否为运算符,`get_priority` 函数用于获取运算符的优先级。`calculate` 函数用于计算两个数字之间的运算结果。`evaluate` 函数是主要的求值函数,它按照算法步骤逐个读取字符,并根据不同情况进行入栈、出栈和计算操作。最后,主函数读取用户输入的算术表达式,并调用 `evaluate` 函数进行求值,并输出结果。
下面是一个测试结果的示例:
```
Enter an arithmetic expression: (1 + 2) * 3 - 4 / 2
Result: 7
```
在该示例中,输入的算术表达式为 `(1 + 2) * 3 - 4 / 2`,求值结果为 `7`。
相关推荐
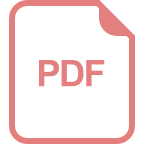
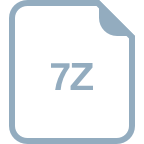
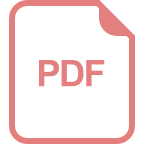
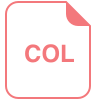
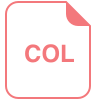
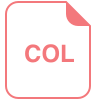
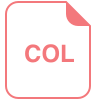
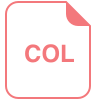









