使用Java编程和springboot框架统计本周第一天 本月第一天 本年第一天起到今天为止的中奖趋势接口 并对接口进行redis缓存处理 过期时间10分钟给出相应的controller层,service层,daoc层及mapper,
时间: 2024-03-10 12:46:43 浏览: 68
好的,以下是一个可能的实现示例,供参考。
1. DAO层及Mapper,这里使用MyBatis框架进行数据库访问,假设中奖记录的表名为`lottery`:
```java
@Mapper
public interface LotteryMapper {
@Select("SELECT COUNT(*) FROM lottery WHERE DATE(create_time) BETWEEN #{startDate} AND #{endDate}")
int countByCreateTime(@Param("startDate") Date startDate, @Param("endDate") Date endDate);
}
```
2. Service层,统计逻辑可以在这里编写,同时使用Redis缓存中间结果:
```java
@Service
public class TrendService {
private final LotteryMapper lotteryMapper;
private final RedisTemplate<String, Object> redisTemplate;
public TrendService(LotteryMapper lotteryMapper, RedisTemplate<String, Object> redisTemplate) {
this.lotteryMapper = lotteryMapper;
this.redisTemplate = redisTemplate;
}
public List<Integer> getTrend() {
String key = "trend";
List<Integer> trend = (List<Integer>) redisTemplate.opsForValue().get(key);
if (trend != null) {
return trend;
}
LocalDateTime now = LocalDateTime.now();
LocalDateTime weekStart = now.with(TemporalAdjusters.previousOrSame(DayOfWeek.MONDAY));
LocalDateTime monthStart = now.withDayOfMonth(1);
LocalDateTime yearStart = now.withDayOfYear(1);
Date start = Date.from(yearStart.atZone(ZoneId.systemDefault()).toInstant());
Date end = Date.from(now.atZone(ZoneId.systemDefault()).toInstant());
int weekCount = lotteryMapper.countByCreateTime(Date.from(weekStart.atZone(ZoneId.systemDefault()).toInstant()), end);
int monthCount = lotteryMapper.countByCreateTime(Date.from(monthStart.atZone(ZoneId.systemDefault()).toInstant()), end);
int yearCount = lotteryMapper.countByCreateTime(start, end);
trend = Arrays.asList(weekCount, monthCount, yearCount);
redisTemplate.opsForValue().set(key, trend);
redisTemplate.expire(key, 10, TimeUnit.MINUTES);
return trend;
}
}
```
3. Controller层,将请求映射到Service方法,并返回统计结果:
```java
@RestController
public class TrendController {
private final TrendService trendService;
public TrendController(TrendService trendService) {
this.trendService = trendService;
}
@GetMapping("/trend")
public List<Integer> getTrend() {
return trendService.getTrend();
}
}
```
需要注意的是,这只是一个简单的示例,具体的实现方式还需要根据您的具体需求进行调整。同时,为了使示例尽可能简单,这里未进行异常处理、日志记录等其他常规操作,实际使用时需要添加相应的处理逻辑。
阅读全文
相关推荐
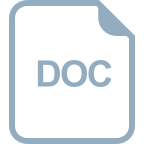
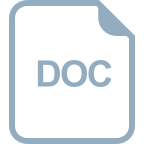
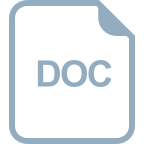







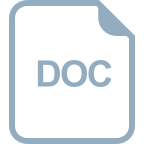
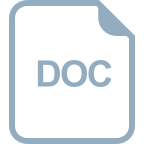
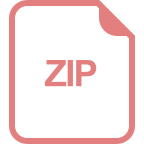
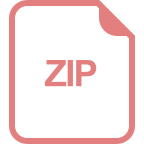
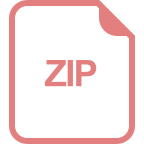
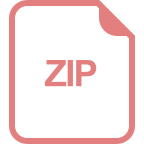
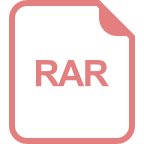
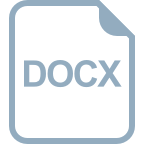