pyqt5标题栏
时间: 2023-06-21 09:13:35 浏览: 170
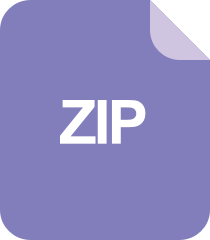
qt5 自定义标题栏

PyQt5是一款Python的GUI编程工具包,用于创建桌面应用程序。如果你想在PyQt5中自定义标题栏,可以通过以下步骤实现:
1. 创建一个QMainWindow对象,并调用setWindowFlag方法来设置窗口样式,例如:
```
self.setWindowFlag(QtCore.Qt.FramelessWindowHint)
```
2. 创建一个QWidget对象作为标题栏,并将其添加到QMainWindow对象的布局中。
3. 在标题栏中添加控件,例如QPushButton、QLabel等。
4. 重写QMainWindow对象的mousePressEvent、mouseMoveEvent和mouseReleaseEvent方法,以实现拖动窗口的功能。
下面是一个简单的例子:
```python
from PyQt5 import QtCore, QtWidgets
class CustomTitleBar(QtWidgets.QWidget):
def __init__(self, parent=None):
super(CustomTitleBar, self).__init__(parent)
self.setParent(parent)
self.setFixedHeight(30)
layout = QtWidgets.QHBoxLayout(self)
layout.setContentsMargins(0, 0, 0, 0)
layout.setSpacing(0)
self.title = QtWidgets.QLabel(self)
self.title.setMargin(5)
layout.addWidget(self.title)
self.btn_close = QtWidgets.QPushButton(self)
self.btn_close.clicked.connect(self.parent().close)
layout.addWidget(self.btn_close)
layout.addStretch()
class MainWindow(QtWidgets.QMainWindow):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
self.setWindowFlag(QtCore.Qt.FramelessWindowHint)
self.setStyleSheet("background-color: white;")
self.title_bar = CustomTitleBar(self)
self.title_bar.title.setText("Custom Title Bar")
self.setCentralWidget(QtWidgets.QTextEdit(self))
self.resize(500, 300)
def mousePressEvent(self, event):
if event.button() == QtCore.Qt.LeftButton:
self.dragPos = event.globalPos() - self.frameGeometry().topLeft()
event.accept()
def mouseMoveEvent(self, event):
if event.buttons() == QtCore.Qt.LeftButton:
self.move(event.globalPos() - self.dragPos)
event.accept()
if __name__ == '__main__':
import sys
app = QtWidgets.QApplication(sys.argv)
w = MainWindow()
w.show()
sys.exit(app.exec_())
```
这个例子中,我们创建了一个CustomTitleBar类作为标题栏,并将其添加到了QMainWindow对象中。我们还重写了QMainWindow对象的mousePressEvent、mouseMoveEvent和mouseReleaseEvent方法,以实现拖动窗口的功能。
在实际开发中,你可以根据需要自定义标题栏的样式和控件,以及实现更复杂的功能,例如最小化、最大化和还原窗口等。
阅读全文
相关推荐
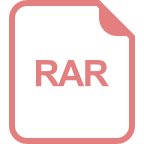



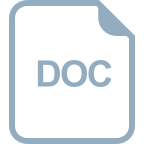











