pyqt5自定义标题栏
时间: 2023-11-08 07:53:15 浏览: 49
您可以使用PyQt5中的QMainWindow和QWidget实现自定义标题栏。下面是一个简单的示例代码,可以实现自定义标题栏:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QMouseEvent
from PyQt5.QtWidgets import QMainWindow, QWidget, QHBoxLayout, QLabel, QPushButton
class CustomTitleBar(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.setStyleSheet("background-color:rgb(38, 38, 38);")
self.setFixedHeight(30)
layout = QHBoxLayout(self)
layout.setContentsMargins(0, 0, 0, 0)
layout.setSpacing(0)
self.title = QLabel(self)
self.title.setStyleSheet("color:white;")
layout.addWidget(self.title, alignment=Qt.AlignCenter)
self.minimize_button = QPushButton("-")
self.minimize_button.clicked.connect(parent.showMinimized)
layout.addWidget(self.minimize_button)
self.close_button = QPushButton("x")
self.close_button.clicked.connect(parent.close)
layout.addWidget(self.close_button)
def mousePressEvent(self, event: QMouseEvent) -> None:
if event.button() == Qt.LeftButton:
self.__mousePressPos = event.pos()
super().mousePressEvent(event)
def mouseMoveEvent(self, event: QMouseEvent) -> None:
if event.buttons() == Qt.LeftButton:
self.parent().move(event.globalPos() - self.__mousePressPos)
super().mouseMoveEvent(event)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Custom Title Bar")
self.setGeometry(100, 100, 500, 500)
self.title_bar = CustomTitleBar(self)
self.setCentralWidget(QWidget(self))
self.centralWidget().setStyleSheet("background-color:rgb(44, 44, 44);")
self.setWindowFlags(Qt.FramelessWindowHint)
layout = QHBoxLayout(self.centralWidget())
layout.setContentsMargins(0, 0, 0, 0)
layout.setSpacing(0)
layout.addWidget(QLabel("Content", self.centralWidget()), alignment=Qt.AlignCenter)
self.show()
def setTitle(self, title):
self.title_bar.title.setText(title)
if __name__ == "__main__":
import sys
from PyQt5.QtWidgets import QApplication
app = QApplication(sys.argv)
window = MainWindow()
window.setTitle("Custom Title Bar")
sys.exit(app.exec_())
```
这段代码中,我们首先定义了一个CustomTitleBar类,用于创建自定义的标题栏。该类继承自QWidget,包含一个QHBoxLayout布局,用于添加标题和最小化、关闭按钮。我们还重载了mousePressEvent和mouseMoveEvent方法,用于实现拖动窗口的功能。
然后,我们创建了一个MainWindow类,继承自QMainWindow。在该类的构造函数中,我们创建了一个CustomTitleBar对象,并将其设置为窗口的标题栏。我们还使用setCentralWidget方法设置了一个QWidget对象作为窗口的中心部件,用于显示窗口的内容。最后,我们使用setWindowFlags方法将窗口的边框设置为无边框。
在主程序中,我们创建了一个QApplication对象和一个MainWindow对象,并设置了窗口的标题。最后,我们调用app.exec_()方法启动应用程序。运行程序后,应该可以看到一个自定义的标题栏和一个灰色的窗口内容区域。
相关推荐
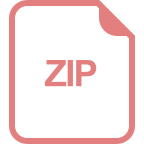














