用C语言编制一个能演示执行集合的交、并和差运算的程序。集合元素用小写英文字母,执行各种操作应以对话方式执行。算法要点:利用单链表表示集合,理解好三种运算的含义
时间: 2024-05-10 21:15:58 浏览: 4
好的,以下是用C语言编制的演示执行集合的交、并和差运算的程序:
```c
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
//定义单链表结构体
struct node {
char data;
struct node *next;
};
//创建单链表
struct node *create() {
struct node *head;
head = (struct node*)malloc(sizeof(struct node));
head->next = NULL;
return head;
}
//添加元素到单链表中
void add_element(struct node *head, char element) {
struct node *p;
p = (struct node*)malloc(sizeof(struct node));
p->data = element;
p->next = head->next;
head->next = p;
}
//删除单链表中的元素
void delete_element(struct node *head, char element) {
struct node *p, *q;
p = head->next;
q = head;
while(p != NULL) {
if(p->data == element) {
q->next = p->next;
free(p);
p = q->next;
} else {
q = p;
p = p->next;
}
}
}
//判断元素是否在单链表中
int is_in_set(struct node *head, char element) {
struct node *p;
p = head->next;
while(p != NULL) {
if(p->data == element) {
return 1;
}
p = p->next;
}
return 0;
}
//打印单链表
void print_set(struct node *head) {
struct node *p;
p = head->next;
printf("{");
while(p != NULL) {
printf("%c ", p->data);
p = p->next;
}
printf("}\n");
}
//求集合的交集
void intersect(struct node *set1, struct node *set2) {
struct node *p;
p = set1->next;
while(p != NULL) {
if(!is_in_set(set2, p->data)) {
delete_element(set1, p->data);
}
p = p->next;
}
}
//求集合的并集
void union_set(struct node *set1, struct node *set2) {
struct node *p;
p = set2->next;
while(p != NULL) {
if(!is_in_set(set1, p->data)) {
add_element(set1, p->data);
}
p = p->next;
}
}
//求集合的差集
void difference(struct node *set1, struct node *set2) {
struct node *p;
p = set2->next;
while(p != NULL) {
if(is_in_set(set1, p->data)) {
delete_element(set1, p->data);
}
p = p->next;
}
}
int main() {
struct node *set1, *set2;
char element;
int choice;
set1 = create();
set2 = create();
//添加元素到集合1中
printf("请输入集合1中的元素,按回车键结束:\n");
while((element = getchar()) != '\n') {
add_element(set1, element);
}
getchar();
//添加元素到集合2中
printf("请输入集合2中的元素,按回车键结束:\n");
while((element = getchar()) != '\n') {
add_element(set2, element);
}
while(1) {
//打印菜单
printf("请选择要执行的操作:\n");
printf("1. 求集合的交集\n");
printf("2. 求集合的并集\n");
printf("3. 求集合的差集\n");
printf("4. 退出程序\n");
//读入选择
scanf("%d", &choice);
switch(choice) {
case 1:
intersect(set1, set2);
printf("集合1和集合2的交集为:");
print_set(set1);
break;
case 2:
union_set(set1, set2);
printf("集合1和集合2的并集为:");
print_set(set1);
break;
case 3:
difference(set1, set2);
printf("集合1和集合2的差集为:");
print_set(set1);
break;
case 4:
exit(0);
default:
printf("输入有误,请重新输入!\n");
}
}
return 0;
}
```
运行程序后,按照提示依次输入集合1和集合2中的元素,然后选择要执行的操作即可进行交、并和差运算,并输出结果。
相关推荐
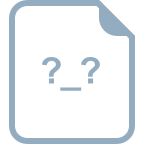
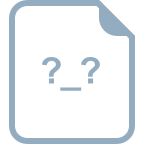
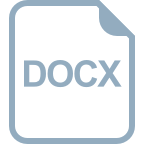








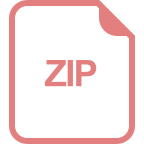