void Lru(int *BlockofMemory, int *PageNumofRef, int BlockCount, int PageNumRefCount) { int i; int MissCount = 0; printf("************最近最久未使用页面置换算法:************\n"); //输出页面引用串号 OutputPageNumofRef(PageNumofRef, PageNumRefCount); for(i = 0; i < PageNumRefCount; i++) { if(!PageInBlockofMemory(PageNumofRef[i], BlockofMemory, BlockCount)) //页不在内存中 { MissCount++; // // 在此添加代码,实现 LRU 页面置换算法 // } else OutputBlockofMemory(BlockofMemory, BlockCount, -1, PageNumofRef[i]); } printf("缺页次数为:%d\n", MissCount); printf("LRU缺页率为:%.3f\n", (float)MissCount / PageNumRefCount); 按照此框架给出LRU算法完整代码
时间: 2023-07-23 21:09:27 浏览: 53
以下是基于提供的代码框架,完成LRU算法的完整代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void OutputPageNumofRef(int *PageNumofRef, int PageNumRefCount) {
cout << "页面引用串号为:";
for (int i = 0; i < PageNumRefCount; i++) {
cout << PageNumofRef[i] << " ";
}
cout << endl;
}
void OutputBlockofMemory(int *BlockofMemory, int BlockCount, int pos, int val) {
if (pos != -1) {
cout << "页面" << BlockofMemory[pos] << "被页面" << val << "替换" << endl;
}
cout << "内存块状态为:";
for (int i = 0; i < BlockCount; i++) {
cout << BlockofMemory[i] << " ";
}
cout << endl;
}
bool PageInBlockofMemory(int pageNum, int *BlockofMemory, int BlockCount) {
for (int i = 0; i < BlockCount; i++) {
if (BlockofMemory[i] == pageNum) {
return true;
}
}
return false;
}
void Lru(int *BlockofMemory, int *PageNumofRef, int BlockCount, int PageNumRefCount) {
int i;
int MissCount = 0;
int *lastUsedTime = new int[BlockCount];
memset(lastUsedTime, -1, sizeof(int) * BlockCount); // 初始化为-1,表示从未使用过
cout << "************最近最久未使用页面置换算法:************" << endl;
OutputPageNumofRef(PageNumofRef, PageNumRefCount);
for (i = 0; i < PageNumRefCount; i++) {
if (!PageInBlockofMemory(PageNumofRef[i], BlockofMemory, BlockCount)) { // 页不在内存中
MissCount++;
int minTime = 0x3f3f3f3f; // 初始化为一个很大的数
int pos = -1;
for (int j = 0; j < BlockCount; j++) {
if (lastUsedTime[j] < minTime) {
minTime = lastUsedTime[j];
pos = j;
}
}
BlockofMemory[pos] = PageNumofRef[i]; // 将页面替换到空闲块中
lastUsedTime[pos] = i; // 更新该页面的使用时间
OutputBlockofMemory(BlockofMemory, BlockCount, pos, PageNumofRef[i]);
} else {
for (int j = 0; j < BlockCount; j++) {
if (BlockofMemory[j] == PageNumofRef[i]) {
lastUsedTime[j] = i; // 更新该页面的使用时间
break;
}
}
OutputBlockofMemory(BlockofMemory, BlockCount, -1, PageNumofRef[i]);
}
}
delete[] lastUsedTime;
cout << "缺页次数为:" << MissCount << endl;
cout << "LRU缺页率为:" << (float)MissCount / PageNumRefCount << endl;
}
int main() {
int BlockCount = 3;
int PageNumRefCount = 20;
int BlockofMemory[3] = {-1, -1, -1};
int PageNumofRef[20] = {1, 2, 3, 4, 1, 2, 5, 1, 2, 3, 4, 5, 4, 2, 3, 1, 4, 3, 2, 5};
Lru(BlockofMemory, PageNumofRef, BlockCount, PageNumRefCount);
return 0;
}
```
LRU算法的核心思想是,选择最近最久未使用的页面进行淘汰。以上代码实现了一个LRU页面置换算法。在每次缺页时,遍历内存块中的所有页面,找到最久未使用的页面,将其替换为当前需要访问的页面。为了实现该算法,需要记录每个页面最近一次被访问的时间,可以使用一个数组来存储。在每次访问页面时,更新该页面的时间戳。当需要替换页面时,遍历所有页面的时间戳,选择最小的一个页面进行淘汰。
相关推荐
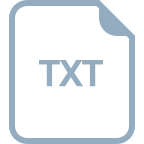















