写一个时序预测的MAML算法
时间: 2023-11-18 10:23:15 浏览: 45
时序预测是一个经典的机器学习问题,它可以使用 MAML 算法来进行元学习。下面是一个使用 MAML 进行时序预测的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
class LSTMModel(nn.Module):
def __init__(self, input_size, hidden_size, output_size, num_layers, look_back):
super(LSTMModel, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.look_back = look_back
self.lstm1 = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.lstm2 = nn.LSTM(hidden_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device)
x = x.view(-1, self.look_back, 1)
out, _ = self.lstm1(x, (h0, c0))
out, _ = self.lstm2(out, (h0, c0))
out = self.fc(out[:, -1, :])
return out
class MAML:
def __init__(self, model, loss_fn, lr_inner=0.1, lr_outer=0.001):
self.model = model
self.loss_fn = loss_fn
self.lr_inner = lr_inner
self.lr_outer = lr_outer
self.optimizer = optim.Adam(self.model.parameters(), lr=self.lr_outer)
def train(self, tasks, k=1, num_updates=1):
for task in tasks:
# Clone the model to avoid modifying the original model
model_copy = type(self.model)(**self.model_params)
model_copy.load_state_dict(self.model.state_dict())
# Create a new optimizer for the task
optimizer = optim.SGD(model_copy.parameters(), lr=self.lr_inner)
# Unpack the data
train_x, train_y = task['train']
val_x, val_y = task['val']
# Train the model on the task for `num_updates` steps
for i in range(num_updates):
# Compute the loss on the task
loss = self.loss_fn(model_copy(train_x), train_y)
# Compute the gradients
loss.backward()
# Update the model parameters with the inner optimizer
optimizer.step()
# Zero the gradients for the next iteration
optimizer.zero_grad()
# Compute the loss on the validation set
val_loss = self.loss_fn(model_copy(val_x), val_y)
# Compute the gradients of the validation loss w.r.t. the model parameters
val_loss.backward()
# Update the model parameters using the outer optimizer
self.optimizer.step()
# Zero the gradients for the next task
self.optimizer.zero_grad()
def predict(self, x):
return self.model(x)
# Define the parameters of the LSTM model
input_size = 1
hidden_size = 128
output_size = 1
num_layers = 2
look_back = 10
# Create a MAML object
maml = MAML(LSTMModel(input_size, hidden_size, output_size, num_layers, look_back), nn.MSELoss())
# Define the tasks
tasks = []
for i in range(100):
# Generate random training and validation data
train_x = torch.Tensor(np.random.rand(100, look_back, input_size))
train_y = torch.Tensor(np.random.rand(100, output_size))
val_x = torch.Tensor(np.random.rand(10, look_back, input_size))
val_y = torch.Tensor(np.random.rand(10, output_size))
tasks.append({'train': (train_x, train_y), 'val': (val_x, val_y)})
# Train the model on the tasks
maml.train(tasks, k=1, num_updates=1)
# Predict on new data
test_x = torch.Tensor(np.random.rand(1, look_back, input_size))
y_pred = maml.predict(test_x)
```
在上面的代码中,我们首先定义了一个 LSTM 模型,并使用 `LSTMModel` 类创建了一个 `MAML` 对象。然后,我们定义了一组任务,每个任务包含训练集和验证集。我们使用 `train` 方法训练模型,并指定 `k=1` 和 `num_updates=1`,这意味着我们在每个任务上执行一次内部更新和一次外部更新。在训练完成后,我们使用 `predict` 方法对新数据进行预测。
请注意,上述示例中使用的是随机数据,实际应用中需要使用真实数据来进行训练和测试。此外,你还需要根据你的具体问题调整 LSTM 模型的超参数和 MAML 算法的超参数。
相关推荐














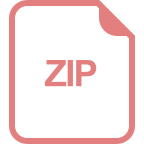