编写如图图形界面,用多线程实现界面上显示10至0的倒计时,要求: (1). 按钮文本初始化为“开始”,点击后变换为“暂停”,倒计时开始,再次点击按钮又变换为“开始”,倒计时暂停; (2). 数字变化间隔为1秒钟; (3). 倒计时为0时,结束程序
时间: 2024-02-22 21:58:22 浏览: 15
好的,我可以为您提供一个使用 Python Tkinter 实现的简单的 GUI 程序,它可以显示一个倒计时计数器,并且可以在不同的线程中启动和暂停倒计时。以下是代码:
```python
import tkinter as tk
import threading
import time
class CountdownTimer:
def __init__(self, master):
self.master = master
master.title("倒计时")
self.countdown_value = tk.StringVar()
self.countdown_value.set("10")
self.countdown_label = tk.Label(master, textvariable=self.countdown_value, font=("Arial", 36))
self.countdown_label.pack(padx=100, pady=50)
self.start_button = tk.Button(master, text="开始", command=self.start_countdown)
self.start_button.pack(pady=10)
self.is_running = False
self.countdown_thread = None
def start_countdown(self):
if not self.is_running:
self.is_running = True
self.start_button.config(text="暂停")
self.countdown_thread = threading.Thread(target=self.countdown)
self.countdown_thread.start()
else:
self.is_running = False
self.start_button.config(text="开始")
def countdown(self):
countdown_value = int(self.countdown_value.get())
while countdown_value > 0:
if not self.is_running:
return
self.countdown_value.set(str(countdown_value))
countdown_value -= 1
time.sleep(1)
self.is_running = False
self.start_button.config(text="开始")
root = tk.Tk()
timer = CountdownTimer(root)
root.mainloop()
```
在这个程序中,我们首先定义了一个名为 `CountdownTimer` 的类,它继承自 `tkinter.Frame`。在 `__init__` 方法中,我们创建了一个标签 `countdown_label`,用于显示当前的倒计时数值,以及一个按钮 `start_button`,用于启动和暂停计数器。我们还定义了一个 boolean 变量 `is_running`,用于跟踪计数器是否正在运行,以及一个线程 `countdown_thread`,用于执行倒计时任务。
在 `start_countdown` 方法中,我们检查 `is_running` 变量的值,如果计数器没有在运行,则将其启动,并将按钮文本设置为“暂停”;否则,如果计数器正在运行,则暂停计数器,并将按钮文本设置为“开始”。
在 `countdown` 方法中,我们使用一个循环来执行倒计时任务。在每次循环中,我们首先检查 `is_running` 变量的值,如果计数器已经被暂停,则返回并退出倒计时任务。否则,我们将当前的倒计时数值显示到标签上,并将倒计时数值减少 1 秒。最后,如果倒计时数值已经减少到 0,我们将 `is_running` 变量设置为 False,以便停止计数器,并将按钮文本设置为“开始”。
最后,在主程序中,我们创建了一个 Tkinter 窗口,并将 `CountdownTimer` 类的实例作为其子组件。然后调用 `mainloop` 方法来启动程序。
相关推荐
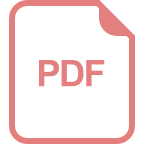
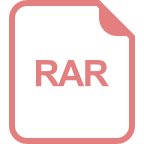














