空间后方交会 c++
时间: 2025-01-08 20:47:55 浏览: 1
### C++ 实现空间后方交会算法
在摄影测量学和计算机视觉领域,空间后方交会(Space Resection)是一个重要的几何计算过程。该方法用于通过已知地面控制点及其对应的图像坐标来求解相机的姿态参数。
#### 空间后方交会原理概述
为了实现这一目标,通常采用最小二乘法优化模型误差,从而估计出最佳的旋转矩阵和平移向量[^1]。具体来说:
- **输入数据**:一组三维世界坐标系下的控制点以及它们在同一张照片上的二维投影位置;
- **输出结果**:描述摄像机相对于固定参照系姿态的外部方位元素——即绕三个轴线的角度变化组成的旋转变换R与沿各方向平动距离构成的位置偏移T;
#### 关键步骤解析
##### 1. 构建观测方程组
对于每一个给定的空间点P(X,Y,Z),其对应于像素平面内的映射关系可以表示为如下形式:
\[ \begin{bmatrix} u \\ v \end{bmatrix}=K[R|t]\begin{bmatrix} X\\ Y\\ Z\\ 1\end{bmatrix}\]
其中\( K=[f_x,0,c_x;0,f_y,c_y]\)代表内参阵列,包含了焦距fx/fy 和主点cx/cy的信息;而\[ R | t ]\)则指代待估测的目标变换矩阵。
##### 2. 应用Levenberg-Marquardt迭代求解最优解
由于实际场景中不可避免存在噪声干扰等因素影响,因此往往借助LM(Levenberg Marquardt)非线性最优化算法来进行数值逼近处理。此过程中不断调整初始猜测值直至达到收敛条件为止。
下面是利用Eigen库完成上述操作的一个简化版代码片段:
```cpp
#include <iostream>
#include <vector>
#include "opencv2/core.hpp"
#include "ceres/ceres.h"
using namespace std;
using namespace cv;
struct ReprojectionError {
ReprojectionError(const Vector3d& point_world,
const Point2d& point_image)
: _point_world(point_world), _point_image(point_image) {}
template<typename T>
bool operator()(const T* const camera_params,// Camera intrinsics and extrinsics as one vector.
T* residuals)const{
Eigen::Matrix<T, 3, 1> p_w(_point_world[0],_point_world[1],_point_world[2]);
Eigen::Map<Eigen::Matrix<T,9,1>> cam(camera_params);
auto r = cam.head<3>();
auto t = cam.segment<3>(3);
// Rodrigues formula to convert rotation vector into matrix form
ceres::AngleAxisToRotationMatrix(r,&p_w,r.data());
// Apply transformation
Eigen::Matrix<T, 3, 1> transformed_point = (r * p_w + t).eval();
// Project onto image plane using intrinsic parameters
double fx=camera_params[6];
double fy=camera_params[7];
double cx=camera_params[8];
double cy=camera_params[9];
T predicted_u=transformed_point.x() / transformed_point.z()*fx+cx;
T predicted_v=transformed_point.y()/transformed_point.z()*fy+cy;
// Compute reprojection error
residuals[0]=predicted_u-T(_point_image.x);
residuals[1]=predicted_v-T(_point_image.y);
return true;
}
private:
Vector3d _point_world;
Point2d _point_image;
};
void solve_space_resection(vector<Point3d>& points_world,vector<Point2d>& points_img){
Problem problem;
static constexpr int NUM_PARAMS = 10; // Rotation (3), Translation (3), Focal Lengths (2), Principal Points (2)
double initial_guess[NUM_PARAMS]={
/*rx*/0.,/*ry*/0.,/*rz*/0.,
/*tx*/0.,/*ty*/0.,/*tz*/0.,
/*fx*/1000.,/*fy*/1000.,
/*cx*/img.cols/2.,/*cy*/img.rows/2.
};
for(size_t i=0;i<points_world.size();i++){
problem.AddResidualBlock(
new AutoDiffCostFunction<ReprojectionError,2,NUM_PARAMS>(
new ReprojectionError(points_world[i],points_img[i])),
nullptr,
initial_guess);
}
Solver::Options options;
options.linear_solver_type=SparseNormalCholesky;
options.minimizer_progress_to_stdout=true;
Solver::Summary summary;
Solve(options,nullptr,&summary);
}
```
这段程序展示了如何定义重投影残差函数并将其加入到`Problem`对象中去构建完整的数学表达式体系结构。最终调用了Ceres Solver提供的接口执行具体的寻优运算流程。
阅读全文
相关推荐



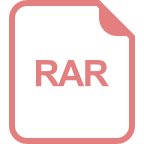


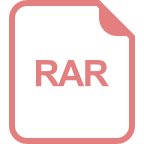









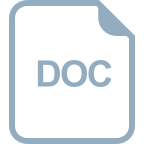
