def img_show()函数
时间: 2024-05-06 10:14:46 浏览: 9
`img_show()` 函数用于显示图片,通常在图像处理和计算机视觉中使用。以下是一个简单的 `img_show()` 函数的例子:
```python
import cv2
def img_show(img_path):
img = cv2.imread(img_path)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个例子中,我们使用 OpenCV 库中的 `imread()` 函数加载图像,并使用 `imshow()` 函数显示图像。然后,我们使用 `waitKey()` 函数等待用户按下任意键,以便继续执行代码。最后,我们使用 `destroyAllWindows()` 函数关闭窗口并释放内存。
要使用这个函数,只需调用 `img_show()` 并传递图像路径作为参数。例如:
```python
img_show('image.jpg')
```
这将在一个新窗口中显示名为 `image.jpg` 的图像。
相关问题
def img_show(name, img):
这是一个 Python 函数,用于在图像窗口中显示给定名称和图像。
参数:
- name:字符串类型,用于给窗口命名。
- img:图像数据,可以是 NumPy 数组或 OpenCV Mat 对象。
示例用法:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 显示图像
img_show('image', img)
# 等待按下任意键
cv2.waitKey(0)
# 关闭窗口
cv2.destroyAllWindows()
```
def mask_on_rgb_image(mask, img, num_class=3): h, w = img.shape[:2] img_show = np.zeros((h, w, 3)) if len(img.shape) == 3: img_show = img else: img = np.expand_dims(img, axis=2) img_show = np.concatenate((img, img, img), axis=-1) # specify color 1:red 2:green 3:blue img_show[np.where(mask == 1)] = [255, 0, 0] img_show[np.where(mask == 2)] = [0, 255, 0] img_show[np.where(mask == 3)] = [0, 0, 255] return img_show
这是一个 Python 函数,用于在彩色图像上根据给定的掩码(mask)展示不同的区域。具体实现方法如下:
- `mask_on_rgb_image(mask, img, num_class=3)`:函数名称,输入参数包括掩码 mask、彩色图像 img 和分类数 num_class,其中 num_class 默认为3。
- `h, w = img.shape[:2]`:获取图像的高度和宽度。
- `img_show = np.zeros((h, w, 3))`:创建一个与 img 大小相同的全0数组,用于展示掩码效果。
- `if len(img.shape) == 3: img_show = img`:如果 img 是彩色图像,直接将 img 赋值给 img_show,否则需要将 img 转换为彩色图像。
- `else: img = np.expand_dims(img, axis=2) img_show = np.concatenate((img, img, img), axis=-1)`:如果 img 是灰度图像,需要将其转换为彩色图像,具体方法是先在第3个维度上扩展为3维,然后将三个维度上的值连接起来。
- `img_show[np.where(mask == 1)] = [255, 0, 0]`:将掩码中等于1的区域赋值为红色,对应的 RGB 值为 [255, 0, 0]。
- `img_show[np.where(mask == 2)] = [0, 255, 0]`:将掩码中等于2的区域赋值为绿色,对应的 RGB 值为 [0, 255, 0]。
- `img_show[np.where(mask == 3)] = [0, 0, 255]`:将掩码中等于3的区域赋值为蓝色,对应的 RGB 值为 [0, 0, 255]。
- `return img_show`:返回展示掩码效果后的彩色图像。
这个函数通常用于图像分割任务中,通过将不同类别的区域用不同颜色标注出来,可以直观地观察模型的分割效果。
相关推荐
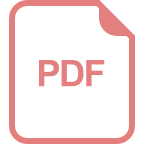
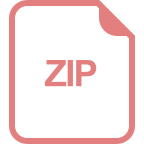
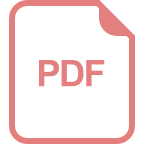













