import cv2 import numpy as np def cv_show(name,img): cv2.imshow(name,img) cv2.waitKey() cv2.destroyAllWindows() def get_img(path1,path2): img1 = cv2.imread(path1) img2 = cv2.imread(path2) #原图像变换为灰度图 img1_gray = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY) img2_gray = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY) return img1,img2,img1_gray,img2_gray def get_info(img1_gray,img2_gray): # 尺度不变特征变换 sift = cv2.SIFT_create() # 关键点以及特征向量计算 kp1, des1 = sift.detectAndCompute(img1_gray, None) kp2, des2 = sift.detectAndCompute(img2_gray, None) kp1 = np.float32([kp.pt for kp in kp1]) kp2 = np.float32([kp.pt for kp in kp2]) return kp1,des1,kp2,des2 def get_match(kp1,kp2,des1,des2): # 特征点交叉检验 bf = cv2.BFMatcher() matches = bf.knnMatch(des1, des2,k=2) idx_pair=[] for m,n in matches: if m.distance<n.distance*0.75: idx_pair.append((m.queryIdx,m.trainIdx)) if len(idx_pair)>4: pt_list1 = np.float32([kp1[i] for (i, _) in idx_pair]) pt_list2 = np.float32([kp1[i] for (_, i) in idx_pair]) H,_ = cv2.findHomography(pt_list2,pt_list1,cv2.RANSAC,4) result = cv2.warpPerspective(img2, H, (img1.shape[1] + img2.shape[1], img1.shape[0])) result[0:img1.shape[0], 0:img1.shape[1]] = img1 return result #main函数 path1='img1.jpg' path2='img2.jpg' img1,img2,img1_gray,img2_gray=get_img(path1,path2) kp1,des1,kp2,des2=get_info(img1_gray,img2_gray) result=get_match(kp1,des1,kp2,des2) cv_show('result',result)
时间: 2024-04-01 18:34:16 浏览: 152
这段代码是一个基于SIFT特征点匹配的图像拼接代码。代码中先读取了两张图片,然后将其转换为灰度图像,并利用SIFT算法提取各自的关键点和特征向量,之后利用BFMatcher进行特征点匹配,再进行交叉检验,得到匹配点对,进而利用findHomography计算出变换矩阵H,最后利用warpPerspective函数将图像2变换到图像1的位置,并将两幅图像拼接在一起。最后使用cv_show函数显示拼接后的图像。
需要注意的是,SIFT算法是一种有专利的算法,如果使用OpenCV 3.4.2及以后的版本,需要安装opencv-contrib-python才能使用SIFT算法。如果没有安装该库,可以使用SURF算法代替SIFT算法进行特征提取。同时,该代码还存在一些问题,比如在利用findHomography计算变换矩阵时,应该将pt_list2放在前面,pt_list1放在后面,否则会导致拼接的结果不正确。此外,在利用warpPerspective函数变换图像时,应该先将变换矩阵H取反,否则得到的结果也会不正确。
相关问题
import numpy as np import cv2 class ColorMeter(object): color_hsv = { # HSV,H表示色调(度数表示0-180),S表示饱和度(取值0-255),V表示亮度(取值0-255) # "orange": [np.array([11, 115, 70]), np.array([25, 255, 245])], "yellow": [np.array([11, 115, 70]), np.array([34, 255, 245])], "green": [np.array([35, 115, 70]), np.array([77, 255, 245])], "lightblue": [np.array([78, 115, 70]), np.array([99, 255, 245])], "blue": [np.array([100, 115, 70]), np.array([124, 255, 245])], "purple": [np.array([125, 115, 70]), np.array([155, 255, 245])], "red": [np.array([156, 115, 70]), np.array([179, 255, 245])], } def __init__(self, is_show=False): self.is_show = is_show self.img_shape = None def detect_color(self, frame): self.img_shape = frame.shape res = {} # 将图像转化为HSV格式 hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV) for text, range_ in self.color_hsv.items(): # 去除颜色范围外的其余颜色 mask = cv2.inRange(hsv, range_[0], range_[1]) erosion = cv2.erode(mask, np.ones((1, 1), np.uint8), iterations=2) dilation = cv2.dilate(erosion, np.ones((1, 1), np.uint8), iterations=2) target = cv2.bitwise_and(frame, frame, mask=dilation) # 将滤波后的图像变成二值图像放在binary中 ret, binary = cv2.threshold(dilation, 127, 255, cv2.THRESH_BINARY) # 在binary中发现轮廓,轮廓按照面积从小到大排列 contours, hierarchy = cv2.findContours( binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE ) if len(contours) > 0: # cv2.boundingRect()返回轮廓矩阵的坐标值,四个值为x, y, w, h, 其中x, y为左上角坐标,w,h为矩阵的宽和高 boxes = [ box for box in [cv2.boundingRect(c) for c in contours] if min(frame.shape[0], frame.shape[1]) / 10 < min(box[2], box[3]) < min(frame.shape[0], frame.shape[1]) / 1 ] if boxes: res[text] = boxes if self.is_show: for box in boxes: x, y, w, h = box # 绘制矩形框对轮廓进行定位 cv2.rectangle( frame, (x, y), (x + w, y + h), (153, 153, 0), 2 ) # 将绘制的图像保存并展示 # cv2.imwrite(save_image, img) cv2.putText( frame, # image text, # text (x, y), # literal direction cv2.FONT_HERSHEY_SIMPLEX, # dot font 0.9, # scale (255, 255, 0), # color 2, # border ) if self.is_show: cv2.imshow("image", frame) cv2.waitKey(1) # cv2.destroyAllWindows() return res if __name__ == "__main__": cap = cv2.VideoCapture(0) m = ColorMeter(is_show=True) while True: success, frame = cap.read() res = m.detect_color(frame) print(res) if cv2.waitKey(1) & 0xFF == ord('q'): break
"red": (0, 255, 255),
"green": (85, 255, 128),
"blue": (170, 255, 128) } 你好!我能够理解你正在询问的是如何使用HSV色彩空间来表示不同的颜色。例如,红色的HSV值为(0,255,255),绿色的HSV值为(85,255,128),蓝色的HSV值为(170,255,128)。
import cv2 import numpy as np def cv_show(name,img): cv2.imshow(name,img) cv2.waitKey() cv2.destroyAllWindows() def get_img(path1,path2): img1 = cv2.imread(path1) img2 = cv2.imread(path2) img1 = cv2.resize(img1, (300, 400)) img2 = cv2.resize(img2, (300, 400)) #原图像变换为灰度图 img1_gray = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY) img2_gray = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY) return img1,img2,img1_gray,img2_gray def get_info(img1_gray,img2_gray): # 尺度不变特征变换 sift = cv2.SIFT_create() # 关键点以及特征向量计算 kp1, des1 = sift.detectAndCompute(img1_gray, None) kp2, des2 = sift.detectAndCompute(img2_gray, None) kp1 = np.float32([kp.pt for kp in kp1]) kp2 = np.float32([kp.pt for kp in kp2]) return kp1,des1,kp2,des2 def get_match(kp1,kp2,des1,des2): # 特征点交叉检验 bf = cv2.BFMatcher() matches = bf.knnMatch(des1, des2,k=2) idx_pair=[] for m,n in matches: if m.distance<n.distance*0.75: idx_pair.append((m.queryIdx,m.trainIdx)) if len(idx_pair)>4: pt_list1 = np.float32([kp1[i] for (i, _) in idx_pair]) pt_list2 = np.float32([kp1[i] for (_, i) in idx_pair]) H,_ = cv2.findHomography(pt_list2,pt_list1,cv.RANSAC,4) result = cv2.warpPerspective(img2, H, (img1.shape[1] + img2.shape[1], img1.shape[0])) result[0:img1.shape[0], 0:img1.shape[1]] = img1 return result #main函数 path1='img1.jpg' path2='img2.jpg' img1,img2,img1_gray,img2_gray=get_img(path1,path2) kp1,des1,kp2,des2=get_info(img1_gray,img2_gray) result=get_match(kp1,des1,kp2,des2) cv_show('result',result)对以上代码debug
这段代码的功能是将两张图片进行拼接,其中使用了SIFT算法进行特征点提取和匹配,以及RANSAC算法计算单应性矩阵。对于代码本身,我看到以下几个问题:
1.第一行的import语句应该写在不同的行中,而不是在同一行中。
2.在函数get_match()中,第12行的cv.RANSAC应该改为cv2.RANSAC。
3.在函数get_match()中,第20行的kp1应该改为kp2。
4.在函数get_match()中,第22行的img2应该改为img1。
5.在主函数中,img1和img2的大小应该保持一致,否则无法进行拼接。
6.在主函数中,应该调用cv_show()函数来显示img1和img2,以便查看它们的内容。
7.在主函数中,应该将result保存为文件,而不是直接展示在屏幕上。
希望我的解答能够帮助到你!
阅读全文
相关推荐
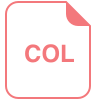
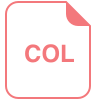
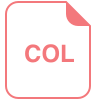
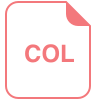
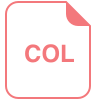
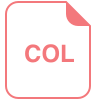
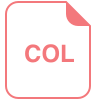
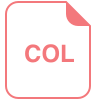
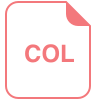
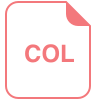
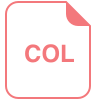
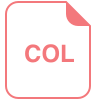
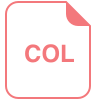



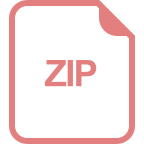