Android 倒计时
时间: 2023-11-21 21:57:39 浏览: 110
Android倒计时是一种常见的功能,可以在很多应用中被使用,比如倒计时闹钟、计时器、倒计时游戏等等。以下是三种实现Android倒计时的方法:
1.使用CountDownTimer类实现倒计时
```java
new CountDownTimer(60000, 1000) {
public void onTick(long millisUntilFinished) {
// 每隔1秒回调一次该方法
textView.setText("倒计时:" + millisUntilFinished / 1000 + "秒");
}
public void onFinish() {
// 倒计时结束时回调该方法
textView.setText("倒计时结束");
}
}.start();
```
2.使用Handler和Runnable实现倒计时
```java
private int count = 60;
private Handler handler = new Handler();
private Runnable runnable = new Runnable() {
@Override
public void run() {
if (count > 0) {
textView.setText("倒计时:" + count + "秒");
count--;
handler.postDelayed(this, 1000);
} else {
textView.setText("倒计时结束");
}
}
};
handler.postDelayed(runnable, 1000);
```
3.使用Timer和TimerTask实现倒计时
```java
private int count = 60;
private Timer timer = new Timer();
private TimerTask timerTask = new TimerTask() {
@Override
public void run() {
if (count > 0) {
textView.setText("倒计时:" + count + "秒");
count--;
} else {
textView.setText("倒计时结束");
timer.cancel();
}
}
};
timer.schedule(timerTask, 0, 1000);
```
阅读全文
相关推荐
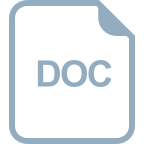
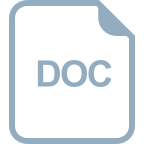
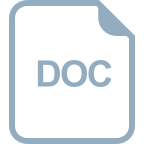
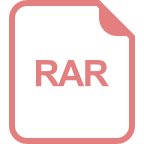
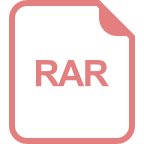
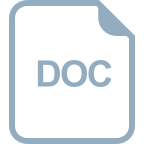
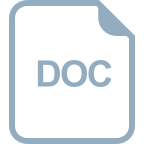
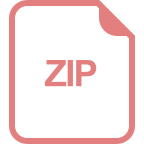
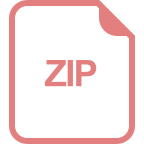
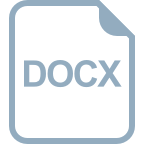
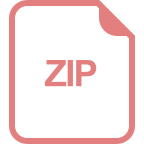
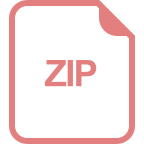
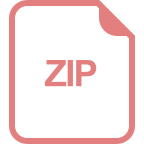
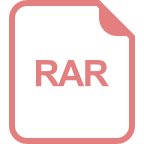