背包问题的分支限界算法实现c++代码
时间: 2024-11-28 20:12:52 浏览: 13
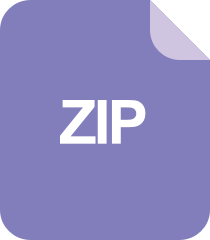
C++分支限界法(BFS求解01背包问题)
背包问题(Knapsack Problem)是一种经典的组合优化问题,分支限界法是一种解决这类问题的搜索策略。以下是基于动态规划和广度优先搜索(BFS)的简单C++实现,用于0-1背包问题:
```cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 定义物品结构
struct Item {
int value;
int weight;
int id; // 物品编号,便于在解集中区分
};
bool operator<(const Item& a, const Item& b) { return a.weight < b.weight; }
// 动态规划函数,计算最大价值
int knapSack(int W, vector<Item>& items, int n) {
vector<vector<int>> dp(n + 1, vector<int>(W + 1, 0));
for (int i = 1; i <= n; ++i) {
for (int w = 1; w <= W; ++w) {
if (items[i - 1].weight <= w) {
dp[i][w] = max(dp[i - 1][w], dp[i - 1][w - items[i - 1].weight] + items[i - 1].value);
} else {
dp[i][w] = dp[i - 1][w];
}
}
}
return dp[n][W];
}
// 分支限界算法
void branchAndBound(vector<Item>& items, int W, int currentWeight, int remainingItems) {
if (remainingItems == 0 || currentWeight > W) {
return;
}
sort(items.begin(), items.end()); // 按照重量从小到大排序
for (Item item : items) {
// 选择加入当前物品
branchAndBound(items, W, currentWeight + item.weight, remainingItems - 1);
// 如果背包还有空间,尝试不加入当前物品
if (currentWeight + item.weight <= W) {
int withoutItem = knapSack(W, items, remainingItems);
int withItem = knapSack(currentWeight + item.weight, items, remainingItems - 1) + item.value;
// 更新最佳解决方案
if (withoutItem < withItem) {
knapSack(W, items, remainingItems) = withoutItem;
}
}
}
}
int main() {
int W = 50; // 包的容量
vector<Item> items = { {60, 10}, {100, 20}, {120, 30} }; // 物品列表
int n = items.size();
cout << "Maximum value in the knapsack: " << knapSack(W, items, n) << endl;
return 0;
}
```
这个程序首先使用动态规划求出不使用分支限界的最优解,然后通过递归和剪枝来尝试各种可能性。`branchAndBound`函数会不断扩展搜索树,直到找到所有可能组合中的最优解。
阅读全文
相关推荐
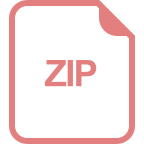
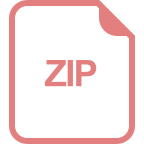



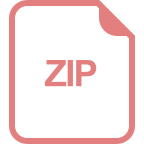
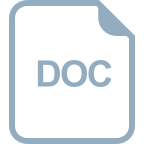
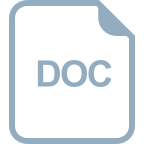
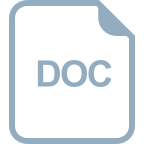








