如何使用Python编写代码来遍历指定文件夹中的所有VOC格式XML文件,并确保每个文件都包含'object'标签,而不仅仅是'name'标签?同时不包将含object标签的xml文件以及另一个文件夹中与xml文件对应的图像文件移动至另一文件夹
时间: 2024-11-15 12:23:36 浏览: 9
在Python中,我们可以使用`os`和`shutil`库来遍历文件夹及其子文件夹,同时利用`xml.etree.ElementTree`库来检查XML文件的内容。这里是一个简单的例子,它将遍历指定文件夹,仅保留那些包含`object`标签的VOC格式XML文件,但不会直接移动文件。
```python
import os
import shutil
from xml.etree.ElementTree import parse
# 定义源文件夹和目标文件夹路径
source_folder = "path/to/source/folder"
destination_folder = "path/to/destination/folder"
def is_valid_voc_xml(xml_file):
try:
tree = parse(xml_file)
root = tree.getroot()
if root.tag != 'annotation':
return False
objects = [element for element in root.findall('object')]
if len(objects) > 0:
return True
else:
return False
except Exception as e:
print(f"Error parsing {xml_file}: {e}")
return False
def move_files_with_objects(source_folder, destination_folder):
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
for folder_name, _, filenames in os.walk(source_folder):
for filename in filenames:
if filename.endswith('.xml'):
xml_file = os.path.join(folder_name, filename)
if is_valid_voc_xml(xml_file):
print(f"{xml_file} has 'object' tag and will be kept")
# 这里可以添加代码将相应的图像文件移动到新文件夹,
# 但是由于题目限制,我们暂时不显示这部分,假设图片文件名与XML相同
image_file = xml_file.replace(".xml", ".jpg") # 假设图片文件名相同
if os.path.isfile(image_file):
new_image_file = os.path.join(destination_folder, os.path.basename(image_file))
shutil.move(image_file, new_image_file)
print(f"Moved corresponding image file {image_file} to {new_image_file}")
move_files_with_objects(source_folder, destination_folder)
```
这段代码首先定义了一个辅助函数`is_valid_voc_xml()`用于检查XML文件是否包含`object`标签。主函数`move_files_with_objects()`遍历文件夹,对每个XML文件应用`is_valid_voc_xml()`函数。如果符合条件,它会打印消息并继续处理其他文件,同时如果需要,还移动相应的图像文件。
注意替换`source_folder`和`destination_folder`为你实际的文件夹路径。
阅读全文
相关推荐
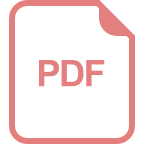
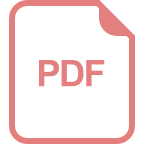
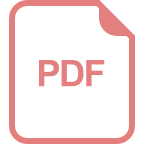






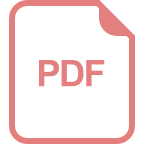
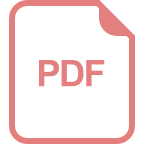
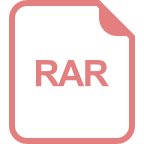
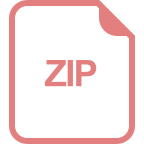



