如何使用Python编写代码来遍历指定文件夹中的所有VOC格式XML文件,并确保每个文件都包含’object’标签,而不仅仅是’name’标签,同时将不包含object标签的xml文件以及与xml文件对应的图像文件移动至另一文件夹
时间: 2024-11-15 09:23:39 浏览: 9
在Python中,你可以使用`os`, `shutil`, 和 `xml.etree.ElementTree`库来完成这个任务。下面是一个示例脚本,它会遍历指定目录及其子目录的所有VOC格式XML文件,并检查它们是否包含`object`标签。如果没有,它会将相应的图片文件和XML文件移动到另一个文件夹。
```python
import os
import shutil
from xml.etree import ElementTree as ET
# 指定源目录和目标文件夹路径
source_dir = '/path/to/source/directory'
target_dir = '/path/to/target/directory'
# 创建目标文件夹,如果不存在
if not os.path.exists(target_dir):
os.makedirs(target_dir)
def check_xml_file(xml_path):
tree = ET.parse(xml_path)
root = tree.getroot()
# 检查是否存在'object'标签
if 'object' in [tag.tag for tag in root.iter()]:
return True
else:
print(f"{xml_path} doesn't contain 'object' tag.")
return False
for dirpath, _, filenames in os.walk(source_dir):
for filename in filenames:
if filename.endswith('.xml') and 'Annotations' in filename: # 排除非VOC XML文件
xml_file = os.path.join(dirpath, filename)
image_file = xml_file.replace('Annotations', '') + '.jpg' # 假设图片文件名与XML类似,只是缺少'Annotations'
if check_xml_file(xml_file):
continue # 如果包含object标签,跳过处理
else:
new_xml_path = os.path.join(target_dir, filename)
new_image_path = os.path.join(target_dir, os.path.basename(image_file))
shutil.move(xml_file, new_xml_path) # 移动XML文件
shutil.move(image_file, new_image_path) # 移动图像文件
阅读全文
相关推荐
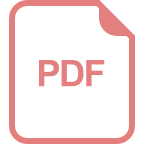
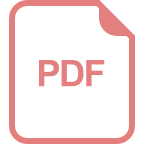
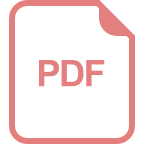






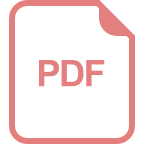
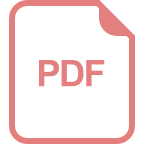
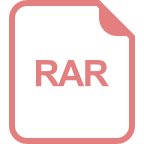
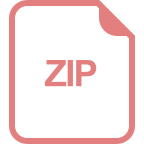




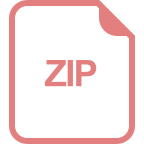