给定一个逻辑表达式,生成真值表对于每一个逻辑表达式,输出其对应的真值表即可。输出的最右边增加了一列,包含了表达式和它的各个值用c语言来写
时间: 2024-03-23 13:38:04 浏览: 22
好的,以下是使用 C 语言实现生成逻辑表达式真值表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include <math.h>
#define MAX_EXPRESSION_LENGTH 100
// 判断字符 c 是否为运算符
bool is_operator(char c) {
return (c == '!' || c == '&' || c == '|');
}
// 判断字符 c 是否为变量
bool is_variable(char c) {
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z');
}
// 计算逻辑表达式 expression 的值
bool evaluate_expression(char *expression, bool *values) {
int len = strlen(expression);
bool stack[len];
int top = -1;
for (int i = 0; i < len; i++) {
char c = expression[i];
if (is_variable(c)) {
int index = c - 'a';
stack[++top] = values[index];
} else if (is_operator(c)) {
if (c == '!') {
bool operand = stack[top--];
stack[++top] = !operand;
} else {
bool operand2 = stack[top--];
bool operand1 = stack[top--];
if (c == '&') {
stack[++top] = operand1 && operand2;
} else {
stack[++top] = operand1 || operand2;
}
}
}
}
return stack[top];
}
// 计算变量的个数
int count_variables(char *expression) {
int count = 0;
int len = strlen(expression);
for (int i = 0; i < len; i++) {
char c = expression[i];
if (is_variable(c)) {
count++;
}
}
return count;
}
// 生成真值表
void generate_truth_table(char *expression) {
int num_variables = count_variables(expression);
bool values[num_variables];
int num_rows = pow(2, num_variables);
printf(" ");
for (int i = 0; i < num_variables; i++) {
printf("%c ", 'a' + i);
}
printf("%s\n", expression);
for (int i = 0; i < num_rows; i++) {
for (int j = 0; j < num_variables; j++) {
values[j] = (i >> (num_variables - j - 1)) & 1;
printf("%d ", values[j]);
}
bool result = evaluate_expression(expression, values);
printf("%d\n", result);
}
}
int main() {
char expression[MAX_EXPRESSION_LENGTH];
printf("请输入一个逻辑表达式:");
fgets(expression, MAX_EXPRESSION_LENGTH, stdin);
expression[strcspn(expression, "\n")] = '\0'; // 去掉末尾的换行符
generate_truth_table(expression);
return 0;
}
```
这段代码首先实现了一个栈数据结构,用于计算逻辑表达式的值。然后,使用 count_variables 函数计算变量的个数,根据变量的个数生成所有可能的变量取值组合,并使用 evaluate_expression 函数计算每个组合下的表达式值。最后,输出真值表的所有行。
注意,这段代码没有进行输入的安全检查,因此请勿输入恶意表达式。
相关推荐
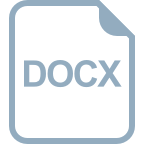
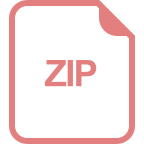















