使用Java Unit test完成下面的代码 void makeForeground() { String NOTIFICATION_CHANNEL_ID = TAG; if (Build.VERSION.SDK_INT < Build.VERSION_CODES.S) { NotificationChannel chan = new NotificationChannel(NOTIFICATION_CHANNEL_ID, NOTIFICATION_CHANNEL_ID, NotificationManager.IMPORTANCE_NONE); chan.setLightColor(Color.BLUE); chan.setLockscreenVisibility(Notification.VISIBILITY_PRIVATE); NotificationManager manager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); manager.createNotificationChannel(chan); NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this, NOTIFICATION_CHANNEL_ID); Notification notification = notificationBuilder.setOngoing(true) .setSmallIcon(R.mipmap.ic_media) .setContentTitle(TAG) .setPriority(NotificationManager.IMPORTANCE_MIN) .setCategory(Notification.CATEGORY_SERVICE) .build(); startForeground(2, notification); } else { //Compatible with Android 12 LogUtils.logI(TAG, "makeForeground : Looking forward to a more comprehensive solution"); } }
时间: 2024-02-14 17:10:28 浏览: 24
你可以使用JUnit来测试这个方法。首先,需要准备好测试所需的对象和依赖项:NotificationChannel,NotificationManager,NotificationCompat.Builder和Notification。这些对象可以使用Mockito框架进行模拟。然后,你可以使用@Before注释来准备测试环境,在测试时调用makeForeground()方法,并使用Mockito.verify()方法来验证方法是否按预期工作。下面是一个可能的测试示例:
```
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.content.Context;
import android.graphics.Color;
import androidx.core.app.NotificationCompat;
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.Mockito;
import org.mockito.MockitoAnnotations;
public class MyServiceTest {
private MyService myService;
@Mock
private NotificationManager notificationManager;
@Mock
private NotificationCompat.Builder notificationBuilder;
@Mock
private Notification notification;
@Before
public void setUp() {
MockitoAnnotations.initMocks(this);
myService = new MyService();
Mockito.when(myService.getSystemService(Context.NOTIFICATION_SERVICE)).thenReturn(notificationManager);
Mockito.when(notificationBuilder.setOngoing(true)).thenReturn(notificationBuilder);
Mockito.when(notificationBuilder.setSmallIcon(Mockito.anyInt())).thenReturn(notificationBuilder);
Mockito.when(notificationBuilder.setContentTitle(Mockito.anyString())).thenReturn(notificationBuilder);
Mockito.when(notificationBuilder.setPriority(Mockito.anyInt())).thenReturn(notificationBuilder);
Mockito.when(notificationBuilder.setCategory(Mockito.anyString())).thenReturn(notificationBuilder);
Mockito.when(notificationBuilder.build()).thenReturn(notification);
}
@Test
public void testMakeForeground() {
// Call the method under test
myService.makeForeground();
// Verify that the correct NotificationChannel was created
Mockito.verify(notificationManager).createNotificationChannel(Mockito.argThat(channel -> {
return channel.getId().equals(MyService.TAG)
&& channel.getName().equals(MyService.TAG)
&& channel.getImportance() == NotificationManager.IMPORTANCE_NONE
&& channel.getLightColor() == Color.BLUE
&& channel.getLockscreenVisibility() == Notification.VISIBILITY_PRIVATE;
}));
// Verify that the correct Notification was built and started
Mockito.verify(notificationManager).startForeground(2, notification);
}
}
```
在这个测试中,我们使用了@Mock注释来创建模拟对象,并使用Mockito.when()方法来设置它们的行为。在setUp()方法中,我们还模拟了MyService.getSystemService()方法的返回值,以便可以使用模拟的NotificationManager对象。在testMakeForeground()方法中,我们调用makeForeground()方法,并使用Mockito.verify()方法来验证预期行为。首先,我们验证正确的NotificationChannel是否被创建,然后验证正确的Notification是否被构建并启动。
相关推荐
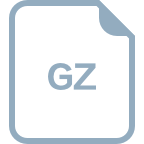














