帮我写字符串压缩的c代码
时间: 2024-09-30 09:13:17 浏览: 26
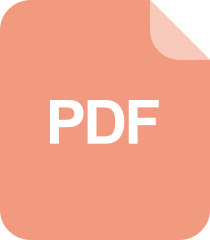
C语言字符串快速压缩算法代码
当然可以,字符串压缩是一种常见的数据结构优化技巧,用于减少存储空间。这里是一个简单的LZW压缩算法的C语言实现示例,它不是标准的文本压缩算法,但可以作为基础理解:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 256
// 定义LZW表
typedef struct {
char data[TABLE_SIZE];
int index[TABLE_SIZE];
} LZWTable;
// 创建新的LZW表
LZWTable* createLZWTable() {
LZWTable *table = (LZWTable*)malloc(sizeof(LZWTable));
memset(table->data, 0, TABLE_SIZE);
for (int i = 0; i < TABLE_SIZE; ++i) {
table->index[i] = i;
}
return table;
}
// 检查并返回对应字符的索引,如果不存在则添加到表中并返回新值
int findOrAdd(char c, LZWTable *table) {
if (table->data[c] == 0) {
table->data[c] = table->index[TABLE_SIZE - 1]++;
return table->index[TABLE_SIZE - 1];
}
return table->data[c];
}
// 压缩字符串
void compressString(char input[], int compressed[], int *compressedLength, LZWTable *table) {
int code = 0;
int prevCode = 0;
*compressedLength = 0;
// 将输入字符串转换成连续的字符编码
for (int i = 0; input[i]; ++i) {
int currentCode = findOrAdd(input[i], table);
if (currentCode != prevCode) { // 新的字符序列
compressed[*compressedLength++] = prevCode << 1 | 1; // 添加前缀1表示开始一个新的序列
}
compressed[*compressedLength++] = currentCode; // 添加当前字符的编码
prevCode = currentCode;
}
// 如果最后一个字符序列没变,不需要额外添加1
if (prevCode != 0) {
compressed[*compressedLength++] = prevCode << 1;
}
}
int main() {
char input[] = "abacabad";
int compressed[length], compressedLength = 0;
LZWTable *table = createLZWTable();
compressString(input, compressed, &compressedLength, table);
printf("Original String: %s\n", input);
printf("Compressed String: ", "");
for (int i = 0; i < compressedLength; ++i) {
printf("%d", compressed[i]);
}
return 0;
}
```
这个例子中,我们首先创建了一个LZW表,然后遍历输入字符串,将相邻的重复字符序列替换为其对应的编码。注意这只是一个简化版的实现,并非高效的压缩算法。
阅读全文
相关推荐
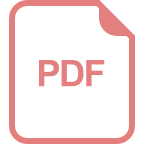
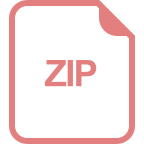















