transforms.ToTensor()在tensorflow下如何替换
时间: 2024-09-21 16:01:26 浏览: 25
在TensorFlow中,`transforms.ToTensor()`是一个PyTorch库中的函数,用于将图像数据转换为张量,并将其像素值从0-255范围归一化到0-1之间。如果你需要在TensorFlow中实现类似的功能,可以使用`tf.image.convert_image_dtype`函数。
例如:
```python
import tensorflow as tf
def to_tensor(image):
image = tf.cast(image, tf.float32) / 255.0
return image
# 使用示例
image_path = "your_image.jpg"
image = tf.io.read_file(image_path)
image = tf.image.decode_jpeg(image, channels=3)
tensor_image = to_tensor(image)
```
这个`to_tensor`函数首先将图像数据从uint8类型转换为float32类型,然后除以255将像素值缩放到0到1之间,达到与PyTorch `ToTensor`类似的效果。
相关问题
transforms.ToPILImage()在tensorflow下如何替换
在TensorFlow中,`transforms.ToPILImage()` 这个来自PyTorch的图像处理函数用于将张量转换成Pillow库中的PIL Image对象。然而,由于TensorFlow本身并不直接支持PIL Image,你需要通过第三方库如`imageio`或`pillow-tensorflow`来实现类似的功能。
如果你想要将一个Tensor转换为PIL Image,可以尝试使用`imageio.imwrite`配合`PIL.Image.fromarray()`,或者使用`tfio.image.decode_image`读取图像数据后转为PIL:
```python
import tensorflow as tf
from PIL import Image
import io
# 假设你有一个Tensor
tensor = ... # TensorFlow image tensor
# 通过imageio库
with io.BytesIO() as buffer:
tensor.numpy().tofile(buffer)
pil_image = Image.open(buffer)
# 或者使用tfio
pil_image = tf.io.decode_image(tensor).numpy().astype('uint8').tolist()[0]
pil_image = Image.fromarray(pil_image)
# 如果需要保存到文件
pil_image.save("output.png")
tf.parse_single_example在mindspore对应的api
在MindSpore中,类似于TensorFlow中的tf.parse_single_example的API是mindspore.dataset.transforms.py_transforms.ParseExample。该API可以从一个TFRecord格式的数据中解析出一个Example对象,然后可以通过Example对象的方法获取其中的features。例如,可以通过Example对象的feature方法获取图片内容、标签等。以下是使用MindSpore中的ParseExample API解析TFRecord数据的示例代码:
```python
import mindspore.dataset as ds
import mindspore.dataset.transforms.py_transforms as py_transforms
# 定义TFRecord数据集路径
tfrecord_path = "train.tfrecord"
# 定义解析Example的函数
def parse_tfrecord(example):
feature_description = {
'image': tf.io.FixedLenFeature([], tf.string),
'label': tf.io.FixedLenFeature([], tf.int64),
}
example = tf.io.parse_single_example(example, feature_description)
return example['image'], example['label']
# 定义MindSpore数据集
dataset = ds.TFRecordDataset(tfrecord_path)
# 定义数据集变换
decode_op = py_transforms.Compose([py_transforms.Decode(), py_transforms.Resize((224, 224))])
parse_op = py_transforms.Compose([py_transforms.ToTensor()])
# 解析Example并进行数据集变换
dataset = dataset.map(operations=parse_tfrecord, input_columns=["example"])
dataset = dataset.map(operations=decode_op, input_columns=["image"])
dataset = dataset.map(operations=parse_op, input_columns=["image", "label"])
```
相关推荐
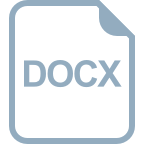
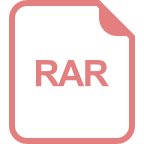
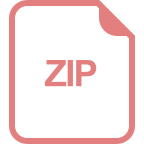













