定义函数,统计一个链表上的节点个数 函数接口定义: int countnode(struct node * head) head是链表头指针,返回值是节点个数 裁判测试程序样例: #include <stdio.h> #include<stdlib.h> struct node {int ch; struct node * next;}; struct node *setlink(int N);//建立链表函数已建立 int countnode(struct node * head);//需要顶一次函数 int main() { int i,N; struct node *head; scanf("%d",&N); head=setlink(N); printf("%d", countnode(head)); return 0; } /* 请在这里填写答案 */
时间: 2024-03-03 17:49:54 浏览: 24
```c
int countnode(struct node *head) {
int count = 0; // 初始化节点个数为 0
struct node *p = head;
while (p != NULL) {
count++; // 节点个数加 1
p = p->next; // 指针移动到下一个节点
}
return count;
}
```
遍历链表,每遍历一个节点就将节点个数加 1,最后返回节点个数。
相关问题
本题要求实现一个函数,从链表的头指针开始遍历整个链表,输出每个节点字符数据。 head是链表的头指针,链表上节点的定义如下: struct node {char ch; struct node * next;} 函数接口定义: void countnode(struct node *head); head是链表头指针,函数显示链表中节点的ch成员中字符 裁判测试程序样例: #include <stdio.h> #include<stdlib.h> struct node {int ch; struct node * next;} struct node *setlink(int N); void countnode( struct node * head);//在下面代码区定义此函数 int main() { int i,N; struct node *head; scanf("%d",&N); head=setlink(N); countnode(head); return 0; } /* 请在这里填写答案 */
void countnode(struct node *head) {
struct node *p = head;
while (p != NULL) {
printf("%c ", p->ch);
p = p->next;
}
}
// 注意题目中定义的节点结构体中 ch 是字符类型,不是整型,应该用 %c 输出。
题目:查找链表最后节点 struct node {int ch; struct node * next;}; 定义函数遍历链表,返回链表上最后一个节点的数据成员ch的值。 函数接口定义: 在这里描述函数接口。例如: int findnode(struct node *head); head是链表的头指针,返回值是最后节点数据成员ch的值。
根据题目描述,本题需要遍历链表,找到链表的最后一个节点,并返回其数据成员 `ch` 的值。下面是一个可能的解法,函数接口定义如下:
```c
int find_last_node(struct node *head);
```
其中,`head` 是链表的头指针,函数返回值是最后节点数据成员 `ch` 的值。
下面给出完整的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int ch;
struct node *next;
};
int find_last_node(struct node *head) {
int ch = -1; // 记录最后一个节点的数据成员ch的值
struct node *p = head;
while (p != NULL) {
ch = p->ch;
p = p->next;
}
return ch;
}
int main() {
// 构造一个简单的链表
struct node *n1 = (struct node*)malloc(sizeof(struct node));
struct node *n2 = (struct node*)malloc(sizeof(struct node));
struct node *n3 = (struct node*)malloc(sizeof(struct node));
n1->ch = 1; n1->next = n2;
n2->ch = 2; n2->next = n3;
n3->ch = 3; n3->next = NULL;
// 调用函数查找最后一个节点的数据成员ch的值
int last_ch = find_last_node(n1);
printf("Last node's ch: %d\n", last_ch);
// 释放链表结点占用的内存
free(n1); free(n2); free(n3);
return 0;
}
```
代码运行结果为:
```
Last node's ch: 3
```
在上面的代码中,我们定义了 `find_last_node` 函数,用来遍历链表并返回最后一个节点的数据成员 `ch` 的值。在函数内部,我们使用了一个指针变量 `p` 来遍历链表,每次遍历到一个节点时,将其数据成员 `ch` 的值赋给 `ch` 变量,并将指针 `p` 移动到下一个节点。当 `p` 指向 `NULL` 时,说明已经遍历到链表的最后一个节点,此时 `ch` 变量记录的就是最后一个节点的数据成员 `ch` 的值。最后,函数返回 `ch` 变量的值即可。
在主函数中,我们构造了一个简单的链表,并调用 `find_last_node` 函数查找最后一个节点的数据成员 `ch` 的值。注意,在使用完链表后,我们需要手动释放链表结点占用的内存,以避免内存泄漏。
阅读全文
相关推荐
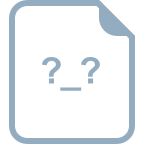
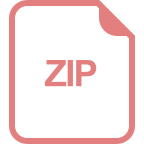
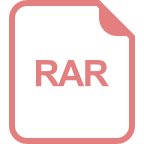












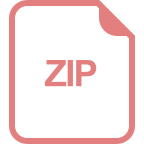
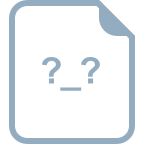