用python完成一段多元线性回归
时间: 2024-01-26 11:43:43 浏览: 22
以下是用Python实现多元线性回归的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义训练数据集
X = np.array([[1, 2, 3],
[1, 3, 4],
[1, 4, 5],
[1, 5, 6]])
y = np.array([[6], [9], [12], [15]])
# 定义模型参数
theta = np.array([[1], [1], [1]])
# 定义代价函数
def compute_cost(X, y, theta):
m = len(y)
J = np.sum((X.dot(theta) - y) ** 2) / (2 * m)
return J
# 定义梯度下降函数
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
J_history = np.zeros((num_iters, 1))
for i in range(num_iters):
theta = theta - alpha / m * X.T.dot(X.dot(theta) - y)
J_history[i] = compute_cost(X, y, theta)
return theta, J_history
# 将特征矩阵和目标向量合并
data = np.hstack((X, y))
# 将数据集随机打乱
np.random.shuffle(data)
# 将数据集分为训练集和测试集
X_train = data[:3, :-1]
y_train = data[:3, -1:]
X_test = data[3:, :-1]
y_test = data[3:, -1:]
# 在训练集上训练模型
theta, J_history = gradient_descent(X_train, y_train, theta, 0.1, 1000)
# 在测试集上测试模型
y_pred = X_test.dot(theta)
# 绘制代价函数随迭代次数的变化曲线
plt.plot(J_history)
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.show()
# 打印模型参数和预测结果
print('theta:', theta)
print('y_pred:', y_pred)
```
在以上代码中,我们首先定义了训练数据集X和目标向量y,然后定义了模型参数theta,代价函数compute_cost和梯度下降函数gradient_descent。
接着,我们将特征矩阵和目标向量合并为一个数据集data,并将其随机打乱,然后将数据集分为训练集和测试集。
在训练集上训练模型,得到模型参数theta和代价函数随迭代次数的变化曲线J_history。
在测试集上测试模型,得到预测结果y_pred,并将其打印出来。
最后,我们使用matplotlib库绘制代价函数随迭代次数的变化曲线。
相关推荐
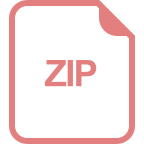














