b.csv文件部分如下: 日期,天气状况,最高气温,最低气温,风力 2023/2/1,阴,2,1,3 2023/2/2,多云,2,1,1 2023/2/3,阴,2,1,2 2023/2/4,阴,2,1,1 2023/2/5,中雨,2,1,2 2023/2/6,小雨,2,1,3 2023/2/7,小雨,1,1,2 2023/2/8,阴,1,1,3 2023/2/9,晴,1,1,2 2023/2/10,晴,2,1,4 2023/2/11,多云,2,1,3 2023/2/12,中雨,3,1,2 2023/2/13,多云,2,1,4 2023/2/14,多云,2,1,1 2023/2/15,多云,2,1,4 2023/2/16,多云,3,1,3 请写出ID3的python代码(根据最高气温,最低气温,风力来预测天气状况)
时间: 2023-08-13 22:02:21 浏览: 27
首先需要加载数据集,然后进行预处理。这里使用pandas库来读取csv文件,然后将数据集划分为训练集和测试集。
```python
import pandas as pd
from sklearn.model_selection import train_test_split
# 加载数据集
data = pd.read_csv('b.csv')
# 将数据集划分为训练集和测试集
train_data, test_data = train_test_split(data, test_size=0.2, random_state=42)
```
接下来,我们需要实现树的节点类,其中包括节点的属性和方法。
```python
class Node:
def __init__(self, feature, label=None, branches=None):
self.feature = feature # 节点的特征
self.label = label # 节点的标签
self.branches = branches or {} # 节点的分支
def add_branch(self, value, node):
self.branches[value] = node
```
然后实现ID3算法,其中包括计算信息熵、计算信息增益、选择最优特征、构建决策树等步骤。
```python
import math
# 计算信息熵
def entropy(data):
entropy = 0
labels = data['天气状况'].unique()
for label in labels:
p = (data['天气状况'] == label).sum() / len(data)
entropy -= p * math.log2(p)
return entropy
# 计算信息增益
def info_gain(data, feature):
base_entropy = entropy(data)
values = data[feature].unique()
new_entropy = 0
for value in values:
sub_data = data[data[feature] == value]
p = len(sub_data) / len(data)
new_entropy += p * entropy(sub_data)
return base_entropy - new_entropy
# 选择最优特征
def best_feature(data, features):
best_feature = None
best_gain = 0
for feature in features:
gain = info_gain(data, feature)
if gain > best_gain:
best_feature = feature
best_gain = gain
return best_feature
# 构建决策树
def build_tree(data, features):
labels = data['天气状况'].unique()
# 如果所有样本都属于同一类别,则直接返回该类别
if len(labels) == 1:
return Node(label=labels[0])
# 如果特征集为空,则直接返回样本中出现最多的类别
if len(features) == 0:
label = data['天气状况'].mode()[0]
return Node(label=label)
# 选择最优特征
best_feature = best_feature(data, features)
# 构建决策树
root = Node(feature=best_feature)
for value in data[best_feature].unique():
sub_data = data[data[best_feature] == value]
sub_tree = build_tree(sub_data, features - {best_feature})
root.add_branch(value, sub_tree)
return root
```
最后,我们可以使用训练集来构建决策树,并使用测试集来验证决策树的准确性。
```python
# 构建决策树
features = set(data.columns) - {'日期', '天气状况'}
tree = build_tree(train_data, features)
# 预测测试集
def predict(tree, sample):
if tree.label is not None:
return tree.label
feature = tree.feature
value = sample[feature]
sub_tree = tree.branches[value]
return predict(sub_tree, sample)
test_data['预测天气状况'] = test_data.apply(lambda x: predict(tree, x), axis=1)
# 计算准确率
accuracy = (test_data['天气状况'] == test_data['预测天气状况']).sum() / len(test_data)
print('准确率:', accuracy)
```
相关推荐
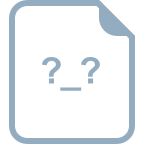
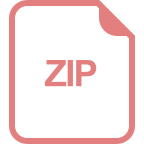









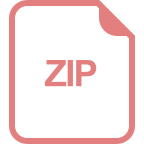
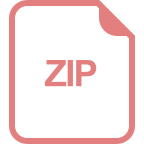
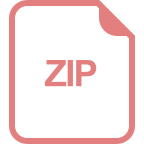
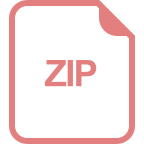
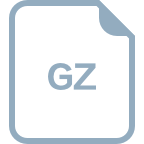