使用vue写一套组件库
时间: 2023-10-24 14:28:12 浏览: 85
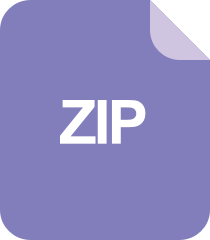
Farris UI Vue 是一套基于Farris Design 的 Vue3 组件库
好的,要使用 Vue 写一套组件库,需要遵循以下步骤:
1. 设计组件:根据项目需求,设计所需的组件样式和功能。
2. 编写组件代码:使用 Vue 组件的基本结构,编写组件的 HTML、CSS 和 JavaScript 代码。
3. 封装组件:将组件的 HTML、CSS 和 JavaScript 代码封装成一个独立的 Vue 组件。
4. 发布组件库:将所有组件打包成一个组件库,发布到 npm 或其他仓库中,供其他开发者使用。
以下是一个简单的步骤示例:
1. 设计组件:假设我们需要设计一个按钮组件,需要考虑按钮的样式、尺寸、颜色等。
2. 编写组件代码:使用 Vue 组件的基本结构,编写组件的 HTML、CSS 和 JavaScript 代码。代码示例:
```html
<template>
<button :class="classes" @click="$emit('click')"><slot></slot></button>
</template>
<script>
export default {
props: {
size: { type: String, default: 'normal' },
type: { type: String, default: 'default' }
},
computed: {
classes () {
return [
'btn',
`btn-${this.type}`,
`btn-${this.size}`
]
}
}
}
</script>
<style scoped>
.btn {
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
font-weight: bold;
}
.btn-default {
background-color: #fff;
color: #333;
}
.btn-primary {
background-color: #007bff;
color: #fff;
}
.btn-success {
background-color: #28a745;
color: #fff;
}
.btn-warning {
background-color: #ffc107;
color: #333;
}
.btn-danger {
background-color: #dc3545;
color: #fff;
}
.btn-normal {
font-size: 16px;
}
.btn-small {
font-size: 14px;
}
.btn-large {
font-size: 18px;
}
</style>
```
3. 封装组件:将组件的 HTML、CSS 和 JavaScript 代码封装成一个独立的 Vue 组件。代码示例:
```javascript
import Button from './Button.vue'
const components = [
Button
]
const install = function (Vue) {
components.forEach(component => {
Vue.component(component.name, component)
})
}
export default {
install,
Button
}
```
4. 发布组件库:将所有组件打包成一个组件库,发布到 npm 或其他仓库中,供其他开发者使用。
以上是一个简单的步骤示例,具体实现还需要根据实际需求进行调整。
阅读全文
相关推荐
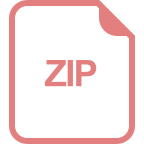
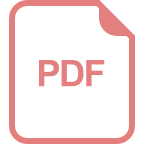















