同一个fragment 怎么切换两个xml布局
时间: 2024-03-16 12:45:26 浏览: 87
你可以在同一个 Fragment 中使用 `LayoutInflater` 来加载不同的布局文件。具体实现方法如下:
1. 在 Fragment 中定义两个布局文件对应的布局 ID,例如 `R.layout.layout1` 和 `R.layout.layout2`。
2. 在 `onCreateView()` 方法中根据不同的条件加载不同的布局文件。
```java
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view;
if (condition1) {
view = inflater.inflate(R.layout.layout1, container, false);
} else {
view = inflater.inflate(R.layout.layout2, container, false);
}
return view;
}
```
这样就可以在同一个 Fragment 中切换不同的布局文件了。
相关问题
同一个fragment 在不重新创建的情况下怎么切换两个xml布局
如果要在不重新创建 Fragment 的情况下切换布局,可以通过 `ViewStub` 来实现。
`ViewStub` 是一个轻量级的视图,它可以被声明在布局文件中但不会立即被加载。当你需要使用它时,它会被填充并替换成你指定的布局文件。
具体实现方法如下:
1. 在 Fragment 的布局文件中添加 `ViewStub`。
```xml
<FrameLayout
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ViewStub
android:id="@+id/stub"
android:layout="@layout/layout1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</FrameLayout>
```
2. 在 Fragment 中定义 `ViewStub` 和两个布局文件对应的布局 ID。
```java
private ViewStub mViewStub;
private View mInflatedView1;
private View mInflatedView2;
```
3. 在 `onCreateView()` 方法中获取 `ViewStub` 并设置它的监听器。
```java
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_layout, container, false);
mViewStub = view.findViewById(R.id.stub);
mViewStub.setOnInflateListener(new ViewStub.OnInflateListener() {
@Override
public void onInflate(ViewStub stub, View inflated) {
if (inflated.getId() == R.id.layout1) {
mInflatedView1 = inflated;
} else {
mInflatedView2 = inflated;
}
}
});
return view;
}
```
4. 在需要切换布局的地方调用 `inflate()` 方法。
```java
if (mInflatedView1 == null) {
mViewStub.setLayoutResource(R.layout.layout1);
mInflatedView1 = mViewStub.inflate();
} else {
mViewStub.setLayoutResource(R.layout.layout2);
mInflatedView2 = mViewStub.inflate();
}
```
这样就可以在同一个 Fragment 中通过 `ViewStub` 来切换不同的布局文件了。
2、创建一个应用程序,设置上下两个布局A、B。B布局内放置两个按钮(text属性的值为ActivityButton1、ActivityButton2)和一个文本框(text属性的值为ActivityTextView)。 (1)在点击两个按钮时,能够动态加载两个Fragment(类名为AFragment、BFragment)到A布局中。 (2)在AFragment中添加按钮(text属性的值为FragmentButton)和文本框(text属性的值为FragmentTextView)。当点击Fragment
Button时,在文本框中显示“Hello Fragment!”的文本。
当然可以!这是一个比较基础的 Android 应用程序,需要用到 Fragment 和布局等知识。我将提供一个简单的示例代码来帮助你完成这个应用程序。
首先,我们需要在布局文件中定义 A 和 B 两个布局:
activity_main.xml:
```xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/layoutA"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
</LinearLayout>
```
activity_b.xml:
```xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/layoutB"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ActivityButton1"/>
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ActivityButton2"/>
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ActivityTextView"/>
</LinearLayout>
```
接下来,我们需要创建两个 Fragment:AFragment 和 BFragment。
AFragment.java:
```java
public class AFragment extends Fragment {
private TextView textView;
public AFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View view = inflater.inflate(R.layout.fragment_a, container, false);
Button button = view.findViewById(R.id.fragmentButton);
textView = view.findViewById(R.id.fragmentTextView);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
textView.setText("Hello Fragment!");
}
});
return view;
}
}
```
BFragment.java:
```java
public class BFragment extends Fragment {
public BFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_b, container, false);
}
}
```
在这两个 Fragment 中,我们分别定义了一个布局文件 fragment_a.xml 和 fragment_b.xml。
fragment_a.xml:
```xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/layoutA"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/fragmentButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="FragmentButton"/>
<TextView
android:id="@+id/fragmentTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="FragmentTextView"/>
</LinearLayout>
```
fragment_b.xml:
```xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/layoutB"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textViewB"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="BFragment"/>
</LinearLayout>
```
最后,我们在 MainActivity 中加载布局并实现按钮的点击事件:
MainActivity.java:
```java
public class MainActivity extends AppCompatActivity {
private LinearLayout layoutA;
private Button button1;
private Button button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
layoutA = findViewById(R.id.layoutA);
button1 = findViewById(R.id.button1);
button2 = findViewById(R.id.button2);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 加载 AFragment
getSupportFragmentManager().beginTransaction()
.replace(R.id.layoutA, new AFragment())
.commit();
}
});
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 加载 BFragment
getSupportFragmentManager().beginTransaction()
.replace(R.id.layoutA, new BFragment())
.commit();
}
});
}
}
```
现在,你可以在 Android Studio 中编译和运行这个应用程序了。当你点击 ActivityButton1 或 ActivityButton2 时,会分别动态加载 AFragment 或 BFragment 到 A 布局中。在 AFragment 中点击 FragmentButton 时,会在文本框中显示“Hello Fragment!”的文本。
希望这个示例代码能够帮助你完成这个应用程序。如果你还有其他问题,欢迎随时问我。
阅读全文
相关推荐
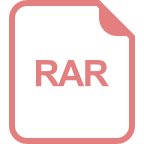
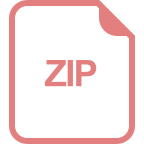
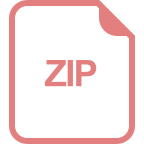
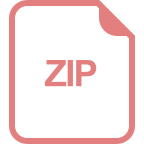
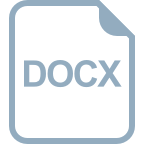
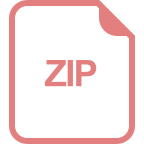
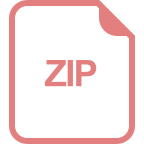
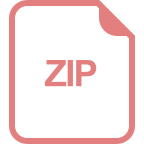
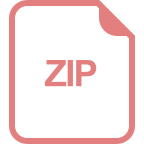
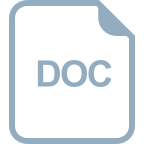





