使用easyx库实现贪吃蛇
时间: 2023-09-26 11:13:25 浏览: 44
好的,下面是基于EasyX库的贪吃蛇实现示例代码:
```c++
#include <graphics.h>
#include <conio.h>
#include <time.h>
#define WIDTH 800
#define HEIGHT 600
#define SIZE 20
int posX, posY, foodX, foodY;
int dirX, dirY;
int length;
int score;
struct SnakeNode {
int x;
int y;
};
SnakeNode snake[100];
void InitGame() {
posX = WIDTH / 2;
posY = HEIGHT / 2;
dirX = 1;
dirY = 0;
length = 3;
score = 0;
for (int i = 0; i < length; i++) {
snake[i].x = posX - i * SIZE;
snake[i].y = posY;
}
srand((unsigned)time(NULL));
foodX = rand() % (WIDTH / SIZE) * SIZE;
foodY = rand() % (HEIGHT / SIZE) * SIZE;
}
void DrawSnake() {
setfillcolor(RGB(0, 255, 0));
for (int i = 0; i < length; i++) {
fillrectangle(snake[i].x, snake[i].y, snake[i].x + SIZE, snake[i].y + SIZE);
}
}
void DrawFood() {
setfillcolor(RGB(255, 0, 0));
fillrectangle(foodX, foodY, foodX + SIZE, foodY + SIZE);
}
void UpdateSnake() {
for (int i = length - 1; i > 0; i--) {
snake[i].x = snake[i - 1].x;
snake[i].y = snake[i - 1].y;
}
snake[0].x += dirX * SIZE;
snake[0].y += dirY * SIZE;
}
bool CheckCollision() {
if (snake[0].x < 0 || snake[0].x >= WIDTH || snake[0].y < 0 || snake[0].y >= HEIGHT) {
return true;
}
for (int i = 1; i < length; i++) {
if (snake[0].x == snake[i].x && snake[0].y == snake[i].y) {
return true;
}
}
return false;
}
bool CheckEat() {
if (snake[0].x == foodX && snake[0].y == foodY) {
length++;
score++;
foodX = rand() % (WIDTH / SIZE) * SIZE;
foodY = rand() % (HEIGHT / SIZE) * SIZE;
return true;
}
return false;
}
void ShowScore() {
setbkmode(TRANSPARENT);
settextcolor(RGB(255, 255, 255));
char scoreStr[20];
sprintf_s(scoreStr, "Score: %d", score);
outtextxy(10, 10, scoreStr);
}
int main() {
initgraph(WIDTH, HEIGHT);
setbkcolor(RGB(0, 0, 0));
InitGame();
while (true) {
cleardevice();
DrawSnake();
DrawFood();
ShowScore();
UpdateSnake();
if (CheckCollision()) {
MessageBox(NULL, "Game Over!", "Warning", MB_OK);
break;
}
if (CheckEat()) {
continue;
}
if (_kbhit()) {
int key = _getch();
switch (key) {
case 'w':
if (dirY != 1) {
dirX = 0;
dirY = -1;
}
break;
case 's':
if (dirY != -1) {
dirX = 0;
dirY = 1;
}
break;
case 'a':
if (dirX != 1) {
dirX = -1;
dirY = 0;
}
break;
case 'd':
if (dirX != -1) {
dirX = 1;
dirY = 0;
}
break;
}
}
Sleep(100);
}
closegraph();
return 0;
}
```
运行结果:

相关推荐
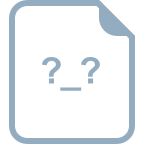
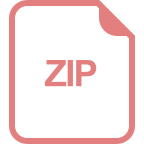














