时间片轮转算法与动态优先权算法java实现
时间: 2023-11-10 11:02:59 浏览: 40
时间片轮转算法的Java实现:
```java
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.Queue;
public class TimeSliceRoundRobin {
public static void main(String[] args) {
int[] arrivalTime = {0, 1, 2, 3};
int[] burstTime = {5, 4, 2, 1};
int timeSlice = 2;
timeSliceRoundRobin(arrivalTime, burstTime, timeSlice);
}
public static void timeSliceRoundRobin(int[] arrivalTime, int[] burstTime, int timeSlice) {
int n = arrivalTime.length;
Queue<Integer> queue = new LinkedList<>();
ArrayList<Integer> waitingTime = new ArrayList<>();
ArrayList<Integer> turnAroundTime = new ArrayList<>();
int time = 0, remainingTime[] = new int[n];
for (int i = 0; i < n; i++) {
remainingTime[i] = burstTime[i];
}
queue.add(0);
while (!queue.isEmpty()) {
int processIndex = queue.poll();
if (remainingTime[processIndex] <= timeSlice) {
time += remainingTime[processIndex];
turnAroundTime.add(time - arrivalTime[processIndex]);
waitingTime.add(time - arrivalTime[processIndex] - burstTime[processIndex]); remainingTime[processIndex] = 0;
} else {
time += timeSlice;
remainingTime[processIndex] -= timeSlice;
}
for (int i = 1; i < n; i++) {
if (remainingTime[i] > 0 && arrivalTime[i] <= time && !queue.contains(i)) {
queue.add(i);
}
}
if (remainingTime[processIndex] > 0) {
queue.add(processIndex);
}
}
int totalWaitingTime = 0, totalTurnAroundTime = 0;
for (int i = 0; i < n; i++) {
totalWaitingTime += waitingTime.get(i);
totalTurnAroundTime += turnAroundTime.get(i);
}
System.out.println("平均等待时间: " + (float) totalWaitingTime / n);
System.out.println("平均周转时间: " + (float) totalTurnAroundTime / n);
}
}
```
动态优先权算法的Java实现:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class DynamicPriority {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入进程个数:");
int n = scanner.nextInt();
int[] arrivalTime = new int[n];
int[] burstTime = new int[n];
int[] priority = new int[n];
for (int i = 0; i < n; i++) {
System.out.print("请输入第" + (i + 1) + "个进程的到达时间:");
arrivalTime[i] = scanner.nextInt();
System.out.print("请输入第" + (i + 1) + "个进程的服务时间:");
burstTime[i] = scanner.nextInt();
System.out.print("请输入第" + (i + 1) + "个进程的优先级:");
priority[i] = scanner.nextInt();
}
dynamicPriority(arrivalTime, burstTime, priority);
}
public static void dynamicPriority(int[] arrivalTime, int[] burstTime, int[] priority) {
int n = arrivalTime.length;
int time = 0;
ArrayList<Integer> waitingTime = new ArrayList<>();
ArrayList<Integer> turnAroundTime = new ArrayList<>();
boolean isCompleted[] = new boolean[n];
int remainingTime[] = new int[n];
int completed = 0;
for (int i = 0; i < n; i++) {
remainingTime[i] = burstTime[i];
}
while (completed != n) {
int processIndex = -1;
int highestPriority = Integer.MIN_VALUE;
for (int i = 0; i < n; i++) {
if (arrivalTime[i] <= time && !isCompleted[i] && priority[i] > highestPriority) {
processIndex = i;
highestPriority = priority[i];
}
}
if (processIndex == -1) {
time++;
} else {
remainingTime[processIndex]--;
time++;
if (remainingTime[processIndex] == 0) {
completed++;
turnAroundTime.add(time - arrivalTime[processIndex]);
waitingTime.add(time - arrivalTime[processIndex] - burstTime[processIndex]);
isCompleted[processIndex] = true;
}
}
}
int totalWaitingTime = 0, totalTurnAroundTime = 0;
for (int i = 0; i < n; i++) {
totalWaitingTime += waitingTime.get(i);
totalTurnAroundTime += turnAroundTime.get(i);
}
System.out.println("平均等待时间: " + (float) totalWaitingTime / n);
System.out.println("平均周转时间: " + (float) totalTurnAroundTime / n);
}
}
```
相关推荐
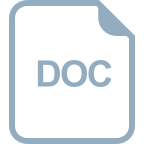
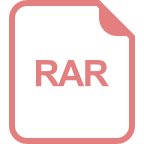














