思路: 首先需要设定游戏的条件和提示,并生成需要猜测的单词或组合。 玩家或电脑每次猜测时,需要输入一个字母或组合,并判断其是否出现在正确答案中。 如果猜测正确,则显示正确的字母或组合,并更新当前猜出的残缺单词或猜测的组合情况。 如果猜测错误,则显示已经猜错的字母,并减少剩余猜错次数。 当剩余猜错次数为0时,游戏结束,显示正确答案并询问是否重新开始游戏。 如果猜测正确,则游戏胜利,显示胜利信息,并询问是否重新开始游戏。 添加是否在玩一次功能,根据玩家的选择决定是否重新开始游戏。c++不使用vector
时间: 2023-12-10 10:41:17 浏览: 223
好的,我可以为您提供一个不使用vector的猜词游戏的代码示例,您可以参考下面的代码实现:
```c++
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_WRONG = 8; // 最大猜错次数
const string WORDS[] = {"COMPUTER", "PROGRAM", "LANGUAGE", "APPLICATION", "NETWORK"}; // 可供猜测的单词
const int WORDS_SIZE = sizeof(WORDS) / sizeof(string); // 单词数量
int main()
{
srand(static_cast<unsigned int>(time(0))); // 用当前时间作为随机数种子
int wrong = 0; // 猜错次数
string target = WORDS[rand() % WORDS_SIZE]; // 随机选择一个单词作为正确答案
string progress(target.size(), '-'); // 初始进度,用横线表示未猜出的字母
string guesses; // 存储已经猜过的字母
cout << "Welcome to the word guessing game. Try to guess the secret word." << endl;
cout << "You can enter one letter or the whole word at a time." << endl;
cout << "You have " << MAX_WRONG << " chances to guess the word." << endl;
// 开始猜词
while (wrong < MAX_WRONG && progress != target)
{
cout << endl << "Progress: " << progress << endl;
cout << "Guesses: " << guesses << endl;
cout << "Enter your guess: ";
string guess;
cin >> guess;
// 判断猜测是否合法
if (guess.size() == 1 || guess.size() == target.size())
{
// 判断猜测是否已经猜过
if (guesses.find(guess) == string::npos)
{
guesses += guess;
// 判断猜测是否正确
if (target.find(guess) != string::npos)
{
cout << "Correct!" << endl;
// 更新进度
for (int i = 0; i < target.size(); ++i)
{
if (target[i] == guess)
{
progress[i] = guess;
}
}
}
else
{
cout << "Wrong!" << endl;
++wrong;
}
}
else
{
cout << "You have already guessed " << guess << ". Please try another one." << endl;
}
}
else
{
cout << "Please enter one letter or the whole word at a time." << endl;
}
}
// 根据猜测结果输出相应信息
if (wrong == MAX_WRONG)
{
cout << endl << "You failed to guess the word. The correct answer is " << target << "." << endl;
}
else
{
cout << endl << "Congratulations! You guessed the word " << target << " correctly!" << endl;
}
// 询问是否重新开始游戏
cout << "Do you want to play again? (y/n)";
char choice;
cin >> choice;
while (choice != 'y' && choice != 'n')
{
cout << "Please enter y or n." << endl;
cin >> choice;
}
// 根据玩家选择决定是否重新开始游戏
if (choice == 'y')
{
main();
}
else
{
cout << "Thank you for playing. Goodbye!" << endl;
}
return 0;
}
```
希望这个代码示例能够对您有所帮助。如果您对代码实现有任何疑问,请随时向我提出。
阅读全文
相关推荐





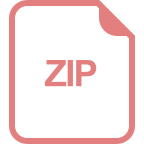












