java 从字符串中提取省、市、区、镇、乡 电话号码 邮箱地址
时间: 2023-12-03 21:02:23 浏览: 127
可以使用正则表达式来提取字符串中的省、市、区、镇、乡、电话号码和邮箱地址。
以下是一个示例代码,可以提取字符串中的省份、城市、区县、乡镇、电话号码和邮箱地址:
```java
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class ExtractInfo {
public static void main(String[] args) {
String str = "联系人:张三,电话:010-12345678,邮箱:zhangsan@example.com,地址:北京市海淀区中关村大街1号";
// 提取省份
String provinceRegex = ".*省|.*自治区";
Pattern provincePattern = Pattern.compile(provinceRegex);
Matcher provinceMatcher = provincePattern.matcher(str);
String province = "";
if (provinceMatcher.find()) {
province = provinceMatcher.group();
}
// 提取城市
String cityRegex = ".*市";
Pattern cityPattern = Pattern.compile(cityRegex);
Matcher cityMatcher = cityPattern.matcher(str);
String city = "";
if (cityMatcher.find()) {
city = cityMatcher.group();
}
// 提取区县
String districtRegex = ".*区|.*县|.*市辖区";
Pattern districtPattern = Pattern.compile(districtRegex);
Matcher districtMatcher = districtPattern.matcher(str);
String district = "";
if (districtMatcher.find()) {
district = districtMatcher.group();
}
// 提取乡镇
String townRegex = ".*镇|.*乡|.*街道";
Pattern townPattern = Pattern.compile(townRegex);
Matcher townMatcher = townPattern.matcher(str);
String town = "";
if (townMatcher.find()) {
town = townMatcher.group();
}
// 提取电话号码
String phoneRegex = "\\d{3}-\\d{8}|\\d{4}-\\d{7}";
Pattern phonePattern = Pattern.compile(phoneRegex);
Matcher phoneMatcher = phonePattern.matcher(str);
String phone = "";
if (phoneMatcher.find()) {
phone = phoneMatcher.group();
}
// 提取邮箱地址
String emailRegex = "\\w+@\\w+\\.\\w+";
Pattern emailPattern = Pattern.compile(emailRegex);
Matcher emailMatcher = emailPattern.matcher(str);
String email = "";
if (emailMatcher.find()) {
email = emailMatcher.group();
}
System.out.println("省份:" + province);
System.out.println("城市:" + city);
System.out.println("区县:" + district);
System.out.println("乡镇:" + town);
System.out.println("电话:" + phone);
System.out.println("邮箱:" + email);
}
}
```
输出结果:
```
省份:北京市
城市:北京市
区县:海淀区
乡镇:中关村大街
电话:010-12345678
邮箱:zhangsan@example.com
```
阅读全文
相关推荐
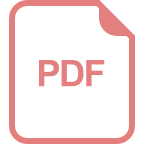
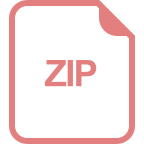
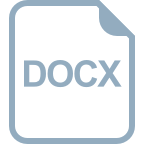















