Python用三角函数
时间: 2024-04-16 11:23:14 浏览: 234
Python中可以使用math模块来进行三角函数的计算。math模块提供了一系列的函数,包括sin、cos、tan等,用于计算三角函数的值。下面是一个简单的示例代码:
```python
import math
# 计算正弦值
x = math.sin(math.pi/2)
print("sin(pi/2) =", x)
# 计算余弦值
y = math.cos(math.pi)
print("cos(pi) =", y)
# 计算正切值
z = math.tan(math.pi/4)
print("tan(pi/4) =", z)
```
运行以上代码,输出结果如下:
```
sin(pi/2) = 1.0
cos(pi) = -1.0
tan(pi/4) = 0.9999999999999999
```
在这个示例中,我们使用了math模块中的sin、cos和tan函数来计算三角函数的值。需要注意的是,这些函数的参数是弧度制的角度。
相关问题
python使用三角函数
在Python中,我们可以利用math模块来进行各种三角函数的运算。math模块提供了大量的数学函数,包括常用的三角函数如正弦(sin)、余弦(cos)、正切(tan)、反正弦(asin)、反余弦(acos)和反正切(atan)等。
下面是一些基本示例:
```python
import math
# 计算角度对应的弧度
angle_degrees = 45
angle_radians = math.radians(angle_degrees)
# 正弦和余弦计算
sin_value = math.sin(angle_radians)
cos_value = math.cos(angle_radians)
# 切线函数计算
tan_value = math.tan(angle_radians)
# 反三角函数计算逆操作
asin_value = math.asin(sin_value)
acos_value = math.acos(cos_value)
atan_value = math.atan(angle_radians) # 如果想计算角度,则需要除以pi并转换单位
print(f"Sin({angle_degrees}°): {sin_value}")
print(f"Cos({angle_degrees}°): {cos_value}")
print(f"Tan({angle_degrees}°): {tan_value}")
print(f"Asin({sin_value}): {asin_value}°")
print(f"Acos({cos_value}): {acos_value}°")
print(f"Atan({angle_radians}): {atan_value}°")
```
python 用三角函数画桃心
可以使用Matplotlib和NumPy库来实现。下面是代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
t = np.arange(0, 2*np.pi, 0.01)
x = 16 * np.sin(t) ** 3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.plot(x, y, color='red')
plt.axis('equal')
plt.show()
```
这段代码会生成一个桃心的图形。其中,`t`是取样点,`x`和`y`是对应点的坐标值,`plt.plot`用于画图,`plt.axis`用于设置坐标轴范围,最后通过`plt.show`显示图形。
阅读全文
相关推荐

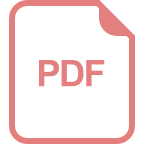








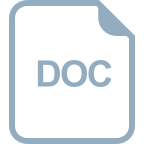
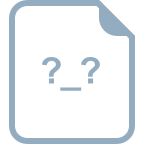
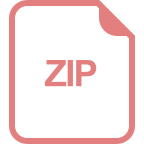