c# codesys modbus rtu
时间: 2023-08-15 19:09:38 浏览: 177
Sure! Here's an example of how you can use C# with CODESYS to communicate over Modbus RTU:
```csharp
using System;
using System.IO.Ports;
namespace ModbusRTUExample
{
class Program
{
static void Main(string[] args)
{
// Modbus RTU serial port settings
string portName = "COM1";
int baudRate = 9600;
Parity parity = Parity.None;
int dataBits = 8;
StopBits stopBits = StopBits.One;
// Create a serial port object
SerialPort port = new SerialPort(portName, baudRate, parity, dataBits, stopBits);
try
{
// Open the serial port
port.Open();
// Modbus RTU message format: slave address + function code + data + CRC16
byte slaveAddress = 1;
byte functionCode = 4;
ushort startingAddress = 0;
ushort numberOfRegisters = 10;
// Build the Modbus RTU message
byte[] message = new byte[8];
message[0] = slaveAddress;
message[1] = functionCode;
message[2] = (byte)(startingAddress >> 8);
message[3] = (byte)startingAddress;
message[4] = (byte)(numberOfRegisters >> 8);
message[5] = (byte)numberOfRegisters;
// Calculate the CRC16 checksum
ushort crc = CalculateCRC(message, 6);
message[6] = (byte)(crc & 0xFF);
message[7] = (byte)(crc >> 8);
// Send the Modbus RTU message
port.Write(message, 0, message.Length);
// Receive the response from the slave device
byte[] response = new byte[1024];
int bytesRead = port.Read(response, 0, response.Length);
// Process the response data
for (int i = 0; i < bytesRead; i++)
{
Console.WriteLine("Byte " + i + ": " + response[i]);
}
}
catch (Exception ex)
{
Console.WriteLine("Error: " + ex.Message);
}
finally
{
// Close the serial port
if (port.IsOpen)
port.Close();
}
Console.ReadLine();
}
static ushort CalculateCRC(byte[] data, int length)
{
ushort crc = 0xFFFF;
for (int i = 0; i < length; i++)
{
crc ^= data[i];
for (int j = 0; j < 8; j++)
{
if ((crc & 0x0001) == 0x0001)
{
crc >>= 1;
crc ^= 0xA001;
}
else
{
crc >>= 1;
}
}
}
return crc;
}
}
}
```
This example shows how to communicate with a Modbus RTU slave device using a serial port connection. You will need to modify the `portName`, `baudRate`, `parity`, `dataBits`, and `stopBits` variables to match your specific serial port settings. Additionally, you can customize the Modbus RTU message by modifying the `slaveAddress`, `functionCode`, `startingAddress`, and `numberOfRegisters` variables. The example also includes a CRC16 calculation function to calculate the checksum for the Modbus RTU message.
阅读全文
相关推荐



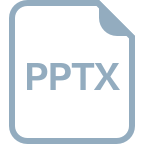
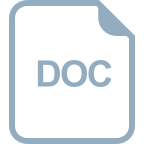
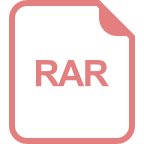
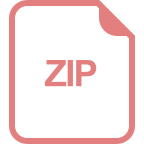
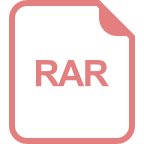
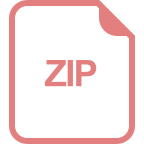
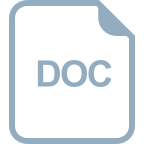
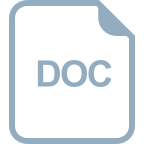


