3.写一个脚本/home/program,要求当给脚本输入参数hello时,脚本返回world,给脚本输入参数world时,脚本返回hello。 而脚本没有参数,屏幕上输出“usage:/home/program hello or world”
时间: 2024-11-06 07:14:29 浏览: 7
为了创建这样一个简单的bash脚本,可以按照下面的方式来编写 `/home/program` 文件:
```bash
#!/bin/bash
# 检查参数数量
if [ "$#" -ne 1 ]; then
echo "usage:/home/program hello or world"
else
case $1 in
hello)
echo "world"
;;
world)
echo "hello"
;;
*)
echo "Invalid input. Please use 'hello' or 'world'."
;;
esac
fi
```
在这个脚本中,`$#` 表示传递给脚本的参数的数量。`case` 结构用于根据参数值执行相应的操作。如果没有提供参数,或提供的不是 "hello" 或 "world",它会给出提示。
运行这个脚本的例子:
- 输入 `./home/program hello`,将得到 "world"。
- 输入 `./home/program world`,将得到 "hello"。
- 输入没有任何参数或者其它无效的参数,将看到 "usage:/home/program hello or world" 的提示。
相关问题
用FAUNC脚本写一个程序
### 回答1:
好的,以下是一个简单的FAUNC脚本程序,它会输出“Hello, world!”:
```
print("Hello, world!")
```
请确保你已经安装了FAUNC解释器,并且将上述代码保存为以“.func”为扩展名的文件,例如“hello.func”。
要运行该程序,请在命令行中输入以下内容:
```
faunc hello.func
```
这将执行hello.func文件中的代码,并输出“Hello, world!”。
### 回答2:
FAUNC是一种自动化测试工具,可以用于编写脚本来执行自动化测试任务。下面是一个用FAUNC脚本写的示例程序。
示例程序的目标是测试一个简单的登录功能。脚本使用FAUNC提供的API对登录表单进行输入、点击登录按钮并验证登录结果。
```
program LoginTest;
procedure Login;
var
usernameInput, passwordInput, loginButton, loginResult: TWebElement;
begin
// 查找用户名输入框元素
usernameInput := Browser.FindWebElement("usernameInput");
// 在用户名输入框中输入用户名
usernameInput.SetText("testUser");
// 查找密码输入框元素
passwordInput := Browser.FindWebElement("passwordInput");
// 在密码输入框中输入密码
passwordInput.SetText("password123");
// 查找登录按钮元素
loginButton := Browser.FindWebElement("loginButton");
// 点击登录按钮
loginButton.Click;
// 等待登录结果元素出现
Browser.WaitUntilWebElementExists("loginResult");
// 查找登录结果元素
loginResult := Browser.FindWebElement("loginResult");
// 验证登录结果
if loginResult.GetText = "登录成功" then
WriteLn("登录测试通过")
else
WriteLn("登录测试失败");
end;
begin
// 打开浏览器
Browser.Open("https://www.example.com");
// 执行登录测试
Login;
// 关闭浏览器
Browser.Close;
end.
```
以上是一个简单的示例程序,使用FAUNC脚本编写的自动化测试脚本来测试登录功能。脚本的主要流程包括输入用户名和密码、点击登录按钮、等待登录结果并验证结果。这个示例程序可以根据实际的测试需求进行修改和扩展。
### 回答3:
FAUNC(Functional Automated uNIt test Creator)是一个自动化测试工具,它基于python语言开发,可以帮助开发人员快速编写、执行、管理和报告自动化测试脚本。
使用FAUNC编写一个程序的步骤如下:
1. 安装FAUNC:首先需要从FAUNC的官方网站(http://www.faunc.org)下载并安装FAUNC框架。
2. 创建测试脚本:在FAUNC的安装目录下,新建一个文件,命名为"test_script.py",用于编写测试脚本。
3. 导入FAUNC相关模块:在测试脚本的开头,导入FAUNC的相关模块,例如:
```
from faunc import TestCase, TestSuite, run
```
4. 创建测试用例类:在测试脚本中,创建一个继承自`TestCase`类的测试用例类,并在其中定义测试方法。例如:
```
class MyTestCase(TestCase):
def test_example(self):
# 测试逻辑代码
pass
```
5. 创建测试套件:在测试脚本中,创建一个`TestSuite`对象,并将测试用例类作为参数传入。例如:
```
suite = TestSuite(MyTestCase)
```
6. 执行测试:在测试脚本的末尾,调用`run()`函数执行测试套件。例如:
```
if __name__ == "__main__":
run(suite)
```
7. 运行测试脚本:保存测试脚本后,在命令行中切换到FAUNC框架的安装目录,并执行以下命令运行脚本:
```
python test_script.py
```
通过以上步骤,你就可以用FAUNC脚本编写一个自动化测试程序了。FAUNC提供了丰富的断言和测试方法,可以用于测试各种功能和场景。编写测试脚本的过程中,你可以根据实际需求,使用FAUNC的API来添加断言、设置测试数据、处理异常等。执行测试后,FAUNC会生成详细的测试报告,方便开发人员查看和分析测试结果。
c#运行python脚本,从c#代码中向脚本中传入参数
在C#中运行Python脚本时,你可以使用`Process.StartInfo`的`Arguments`属性来向Python脚本传递参数。以下是一个示例:
```csharp
using System;
using System.Diagnostics;
class Program
{
static void Main()
{
string pythonPath = "python.exe";
string scriptPath = "path/to/your/script.py";
string argument1 = "Hello";
string argument2 = "World";
ProcessStartInfo startInfo = new ProcessStartInfo();
startInfo.FileName = pythonPath;
startInfo.Arguments = $"{scriptPath} {argument1} {argument2}";
using (Process process = Process.Start(startInfo))
{
process.WaitForExit();
}
Console.WriteLine("Python脚本执行完成!");
}
}
```
在上述示例中,我在`Arguments`属性中使用了字符串插值(string interpolation)来将脚本路径和参数一起传递给Python解释器。在这个例子中,我传递了两个参数`argument1`和`argument2`,并在脚本中可以通过`sys.argv`来获取这些参数。
在你的Python脚本中,你可以使用`sys.argv`来获取传递的参数。以下是一个简单的示例:
```python
import sys
def main():
# 获取传递的参数
argument1 = sys.argv[1]
argument2 = sys.argv[2]
# 在脚本中使用参数
print(f"Argument 1: {argument1}")
print(f"Argument 2: {argument2}")
if __name__ == "__main__":
main()
```
在上述示例中,我使用`sys.argv`来获取传递的参数,并在脚本中打印这些参数。当你运行C#代码时,它将启动Python解释器并将参数传递给脚本,脚本会打印出这些参数。
请确保在C#代码中设置正确的Python解释器路径、脚本路径以及传递的参数。
阅读全文
相关推荐
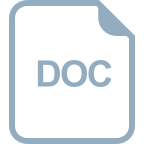
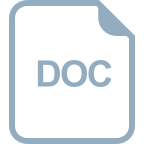
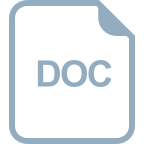
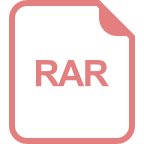
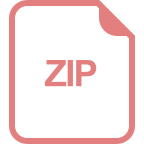
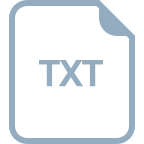
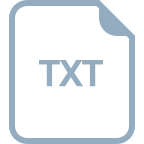
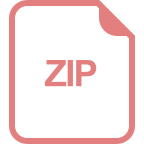
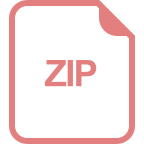
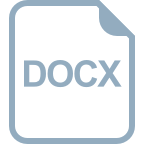
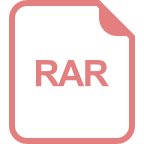
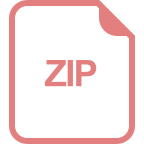
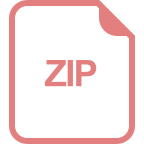
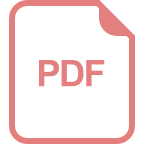
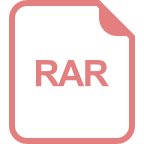
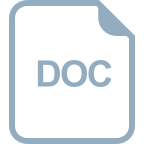
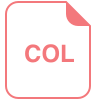