c++opencv 轮廓填充
时间: 2023-06-28 15:16:25 浏览: 108
使用OpenCV进行轮廓填充的基本步骤如下:
1. 读取图像并进行灰度化和二值化处理。
2. 使用 `findContours()` 函数查找轮廓。
3. 对每个轮廓,使用 `drawContours()` 函数进行绘制。
4. 对每个轮廓,使用 `fillPoly()` 函数进行填充。
下面是一个简单的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像并进行灰度化和二值化处理
Mat img = imread("test.png");
Mat gray, binary;
cvtColor(img, gray, COLOR_BGR2GRAY);
threshold(gray, binary, 0, 255, THRESH_BINARY_INV | THRESH_OTSU);
// 使用 findContours() 函数查找轮廓
vector<vector<Point>> contours;
findContours(binary, contours, RETR_LIST, CHAIN_APPROX_SIMPLE);
// 对每个轮廓,使用 drawContours() 函数绘制
Mat draw_img = Mat::zeros(img.size(), CV_8UC3);
for (size_t i = 0; i < contours.size(); i++)
{
drawContours(draw_img, contours, i, Scalar(255, 255, 255), 1, LINE_AA);
}
// 对每个轮廓,使用 fillPoly() 函数进行填充
Mat fill_img = Mat::zeros(img.size(), CV_8UC3);
for (size_t i = 0; i < contours.size(); i++)
{
fillPoly(fill_img, contours, i, Scalar(255, 255, 255), LINE_AA);
}
// 显示结果
imshow("Binary", binary);
imshow("Contours", draw_img);
imshow("Filled", fill_img);
waitKey(0);
return 0;
}
```
在上面的示例代码中,我们使用了 `findContours()` 函数查找轮廓,该函数返回一个 `vector` 类型的容器,其中每个元素都是一个 `vector` 类型的容器,表示一个轮廓。然后,我们可以使用 `drawContours()` 函数绘制轮廓,或者使用 `fillPoly()` 函数对轮廓进行填充。注意,在使用 `fillPoly()` 函数时,必须将轮廓转换为一个 `vector` 类型的容器,其中每个元素表示一个轮廓的顶点集合。
相关推荐
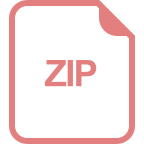
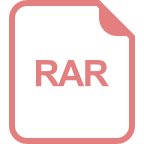
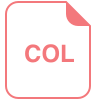
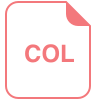
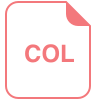
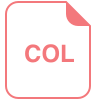
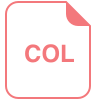









