c++opencv判断轮廓内像素点对比度是否大于阈值
时间: 2023-06-29 07:13:44 浏览: 71
可以按照以下步骤实现:
1. 对轮廓进行填充,生成一个二值图像。
2. 对二值图像进行腐蚀操作,使轮廓内像素点“膨胀”一定程度,避免对比度过低的像素点被误判。
3. 对原始图像进行灰度化。
4. 遍历轮廓内的像素点,计算其像素值与轮廓周围像素值的对比度,判断是否大于阈值。
5. 根据判断结果进行相应的处理。
以下是C++代码示例:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
Mat image = imread("test.jpg"); // 读入原始图像
Mat gray; // 灰度图像
cvtColor(image, gray, COLOR_BGR2GRAY); // 转换为灰度图像
// 提取轮廓
vector<vector<Point>> contours;
findContours(gray, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 遍历每个轮廓
for (int i = 0; i < contours.size(); i++)
{
// 对轮廓进行填充,生成一个二值图像
Mat mask = Mat::zeros(gray.size(), CV_8UC1);
drawContours(mask, contours, i, Scalar(255), FILLED);
// 对二值图像进行腐蚀操作
Mat kernel = getStructuringElement(MORPH_ELLIPSE, Size(5, 5));
erode(mask, mask, kernel);
// 遍历轮廓内的像素点
for (int j = 0; j < contours[i].size(); j++)
{
Point pt = contours[i][j];
// 计算像素点的对比度
double contrast = 0;
for (int k = -1; k <= 1; k++)
{
for (int l = -1; l <= 1; l++)
{
int x = pt.x + k;
int y = pt.y + l;
if (x >= 0 && x < gray.cols && y >= 0 && y < gray.rows && mask.at<uchar>(y, x) == 255)
{
double diff = abs(gray.at<uchar>(pt) - gray.at<uchar>(y, x));
contrast += diff;
}
}
}
contrast /= 9;
// 判断对比度是否大于阈值
double threshold = 20; // 阈值
if (contrast > threshold)
{
// 对比度大于阈值,进行相应的处理
// ...
}
}
}
return 0;
}
```
相关推荐
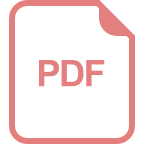














