c++opencv 判断像素点是否在mask区域内
时间: 2023-12-04 12:03:32 浏览: 202
要判断像素点是否在mask区域内,可以使用OpenCV中的cv::bitwise_and函数,将图像与掩膜区域进行按位与操作,得到的结果就是mask区域内的像素点。具体步骤如下:
1.读取图像和掩膜图像:
```
cv::Mat img = cv::imread("image.jpg");
cv::Mat mask = cv::imread("mask.jpg", cv::IMREAD_GRAYSCALE);
```
2.将掩膜图像二值化,使得掩膜图像中的像素值只有0和255:
```
cv::threshold(mask, mask, 128, 255, cv::THRESH_BINARY);
```
3.将图像与掩膜区域进行按位与操作:
```
cv::Mat result;
cv::bitwise_and(img, mask, result);
```
4.判断像素点是否在mask区域内,可以通过查看result中像素点的值来实现。如果像素点的值为0,则不在mask区域内;如果像素点的值不为0,则在mask区域内。
```
int value = result.at<cv::Vec3b>(y, x)[0];
if (value != 0) {
// 像素点在mask区域内
} else {
// 像素点不在mask区域内
}
```
相关问题
c++opencv判断轮廓内像素点对比度是否大于阈值
可以按照以下步骤实现:
1. 对轮廓进行填充,生成一个二值图像。
2. 对二值图像进行腐蚀操作,使轮廓内像素点“膨胀”一定程度,避免对比度过低的像素点被误判。
3. 对原始图像进行灰度化。
4. 遍历轮廓内的像素点,计算其像素值与轮廓周围像素值的对比度,判断是否大于阈值。
5. 根据判断结果进行相应的处理。
以下是C++代码示例:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
Mat image = imread("test.jpg"); // 读入原始图像
Mat gray; // 灰度图像
cvtColor(image, gray, COLOR_BGR2GRAY); // 转换为灰度图像
// 提取轮廓
vector<vector<Point>> contours;
findContours(gray, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 遍历每个轮廓
for (int i = 0; i < contours.size(); i++)
{
// 对轮廓进行填充,生成一个二值图像
Mat mask = Mat::zeros(gray.size(), CV_8UC1);
drawContours(mask, contours, i, Scalar(255), FILLED);
// 对二值图像进行腐蚀操作
Mat kernel = getStructuringElement(MORPH_ELLIPSE, Size(5, 5));
erode(mask, mask, kernel);
// 遍历轮廓内的像素点
for (int j = 0; j < contours[i].size(); j++)
{
Point pt = contours[i][j];
// 计算像素点的对比度
double contrast = 0;
for (int k = -1; k <= 1; k++)
{
for (int l = -1; l <= 1; l++)
{
int x = pt.x + k;
int y = pt.y + l;
if (x >= 0 && x < gray.cols && y >= 0 && y < gray.rows && mask.at<uchar>(y, x) == 255)
{
double diff = abs(gray.at<uchar>(pt) - gray.at<uchar>(y, x));
contrast += diff;
}
}
}
contrast /= 9;
// 判断对比度是否大于阈值
double threshold = 20; // 阈值
if (contrast > threshold)
{
// 对比度大于阈值,进行相应的处理
// ...
}
}
}
return 0;
}
```
c++ opencv 实现保留掩膜中最大区域代码
### 回答1:
在 OpenCV 中,实现保留探测框最大区域的代 码可以通过以下步骤实现:
1. 读取探测框的坐标和大小。
2. 计算探测框的中心点坐标。
3. 遍历图像中所有像素点,计算每个像素点到探测框中心点的距离。
4. 如果像素点到中心点的距离小于探测框宽度的一半,则保留该像素点,否则将其赋值为背景色。
5. 最后得到的结果即为保留探测框最大区域的图像。
下面是示例代码(使用 C++ 实现):
```
#include <opencv2/opencv.hpp>
using namespace cv;
Mat keepLargestRegion(Mat inputImage, Rect boundingBox)
{
// Get bounding box coordinates and size
int x = boundingBox.x;
int y = boundingBox.y;
int width = boundingBox.width;
int height = boundingBox.height;
// Calculate bounding box center
int centerX = x + width / 2;
int centerY = y + height / 2;
// Create output image
Mat outputImage = Mat::zeros(inputImage.size(), CV_8UC1);
// Loop over all pixels in input image
for (int i = 0; i < inputImage.rows; i++) {
for (int j = 0; j < inputImage.cols; j++) {
// Calculate distance between current pixel and bounding box center
double distance = sqrt(pow(i - centerY, 2) + pow(j - centerX, 2));
// If pixel is inside bounding box, copy it to output image
if (distance <= width / 2) {
outputImage.at<uchar>(i, j) = inputImage.at<uchar>(i, j);
}
}
}
return outputImage;
}
```
以上代码中,`inputImage` 表示输入图像,`boundingBox` 表示探测框的位置和大小。函数的返回值是一个新的图像,其中保留了探测框最大区域的像素值。
### 回答2:
下面是使用OpenCV实现保留掩膜中最大区域的示例代码:
```python
import cv2
import numpy as np
# 读取掩膜图像
mask = cv2.imread('mask.png', 0)
# 找到掩膜中的轮廓
contours, hierarchy = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 找到轮廓中的最大区域
max_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_area:
max_area = area
max_contour = contour
# 创建原始图像大小的空白图像
result = np.zeros_like(mask)
# 在空白图像上绘制最大轮廓
cv2.drawContours(result, [max_contour], -1, (255), thickness=cv2.FILLED)
# 显示结果
cv2.imshow('Result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码中,首先读取保留掩膜的图像,然后使用`cv2.findContours`函数找到掩膜中的所有轮廓。接着,遍历所有轮廓,找到面积最大的轮廓,并将其绘制到与掩膜大小相同的空白图像上。最后,显示结果图像。
请注意,以上代码是以Python为例,如果您使用其他编程语言,可以参考OpenCV的官方文档以及对应语言的相关函数来实现相同的功能。
### 回答3:
在OpenCV中实现保留掩膜中最大区域的代码可以通过以下步骤完成:
1. 导入必要的库
```python
import cv2
import numpy as np
```
2. 读取图像并创建掩膜
```python
image = cv2.imread('image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
_, threshold = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV)
```
3. 查找和筛选轮廓
```python
contours, _ = cv2.findContours(threshold, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
largest_contour = max(contours, key=cv2.contourArea)
```
4. 创建新的黑色画布并绘制最大轮廓
```python
mask = np.zeros_like(image)
cv2.drawContours(mask, [largest_contour], -1, (255, 255, 255), thickness=cv2.FILLED)
```
5. 与原始图像进行按位与操作,保留最大区域
```python
result = cv2.bitwise_and(image, mask)
```
6. 显示结果
```python
cv2.imshow('Result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上就是使用OpenCV实现保留掩膜中最大区域的代码。这段代码将读取图像,创建掩膜,找到最大轮廓,绘制轮廓和掩膜,并与原始图像进行按位与操作,最终显示结果。
阅读全文
相关推荐








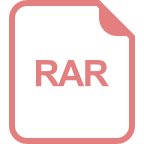





