【C++ OpenCV:图像处理与计算机视觉的利器】:揭秘图像处理算法,从入门到精通
发布时间: 2024-08-07 00:10:00 阅读量: 16 订阅数: 19 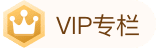
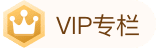
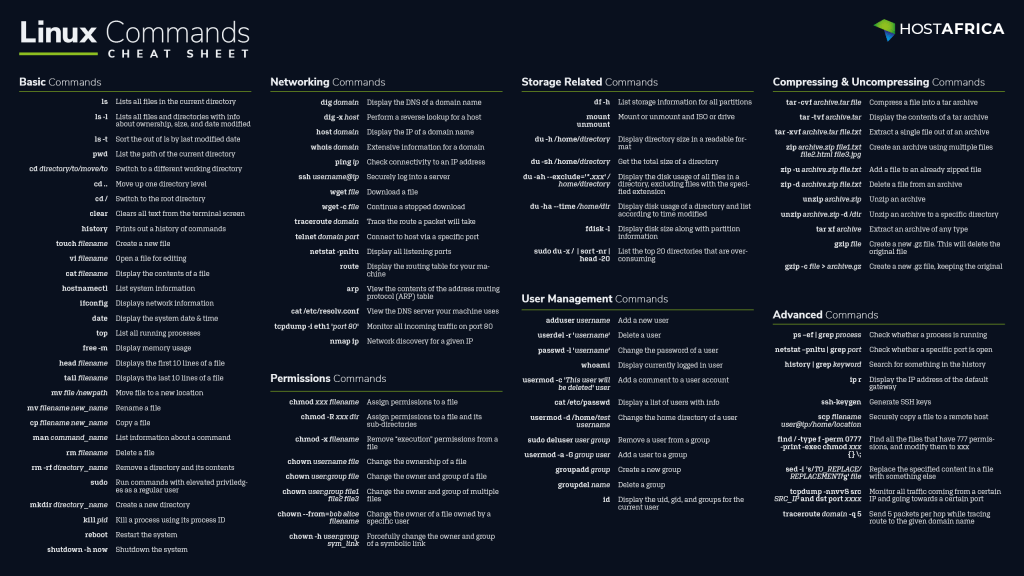
# 1. C++ OpenCV 简介
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉库,为图像处理和计算机视觉算法提供了广泛的功能。它使用 C++ 编写,并支持多种平台,包括 Windows、Linux 和 macOS。
OpenCV 广泛用于各种应用中,包括:
- 图像处理:图像增强、滤波、几何变换
- 计算机视觉:目标检测、图像分割、特征提取
- 机器学习:图像分类、对象识别、人脸检测
# 2. 图像处理基础
### 2.1 图像表示和数据类型
#### 2.1.1 像素、通道和图像尺寸
图像由像素组成,每个像素表示图像中一个点的颜色或强度值。像素通常使用红、绿、蓝(RGB)或灰度值表示。图像的尺寸由宽度和高度以像素为单位定义。
#### 2.1.2 数据类型和图像格式
OpenCV 支持多种图像数据类型,包括:
- `CV_8UC1`:8 位无符号单通道灰度图像
- `CV_8UC3`:8 位无符号三通道 RGB 图像
- `CV_16UC1`:16 位无符号单通道灰度图像
- `CV_32FC1`:32 位浮点单通道灰度图像
图像格式决定了图像数据的存储方式。常见格式包括:
- JPEG:有损压缩格式,适用于照片和图像
- PNG:无损压缩格式,适用于图形和文本
- TIFF:无损格式,适用于高分辨率图像
### 2.2 图像处理基本操作
#### 2.2.1 图像读取、显示和保存
**读取图像**
```cpp
cv::Mat image = cv::imread("image.jpg");
```
**显示图像**
```cpp
cv::imshow("Image", image);
cv::waitKey(0);
```
**保存图像**
```cpp
cv::imwrite("new_image.jpg", image);
```
#### 2.2.2 图像转换和缩放
**图像转换**
```cpp
cv::cvtColor(image, gray_image, cv::COLOR_BGR2GRAY);
```
**图像缩放**
```cpp
cv::resize(image, resized_image, cv::Size(new_width, new_height));
```
#### 2.2.3 图像增强和滤波
**图像增强**
```cpp
cv::equalizeHist(image, equalized_image);
```
**滤波**
```cpp
cv::GaussianBlur(image, blurred_image, cv::Size(kernel_size, kernel_size), sigmaX);
```
# 3.1 图像特征提取
#### 3.1.1 边缘检测和轮廓提取
边缘检测是图像处理中的一项基本技术,它用于检测图像中像素强度的突然变化,从而提取图像中的边缘和轮廓。OpenCV 提供了多种边缘检测算法,包括:
- **Sobel 算子:**使用两个 3x3 滤波器(水平和垂直)来检测水平和垂直边缘。
- **Canny 算子:**一种多阶段算法,包括降噪、梯度计算、非极大值抑制和滞后阈值化。
- **Laplacian 算子:**使用拉普拉斯算子来检测图像中的边缘和斑点。
```cpp
// 使用 Sobel 算子检测边缘
cv::Mat sobel_x, sobel_y;
cv::Sobel(image, sobel_x, CV_16S, 1, 0);
cv::Sobel(image, sobel_y, CV_16S, 0, 1);
```
#### 3.1.2 直方图和颜色空间
直方图是图像中像素值分布的统计表示。它可以用于分析图像的亮度、对比度和颜色分布。OpenCV 提供了多种颜色空间,包括:
- **RGB:**红、绿、蓝三原色空间。
- **HSV:**色调、饱和度、明度空间。
- **YCrCb:**亮度、色度差、色度差空间。
```cpp
// 计算图像的直方图
cv::MatND hist;
int channels[] = {0};
int histSize[] = {256};
float hranges[] = {0, 256};
cv::calcHist(&image, 1, channels, cv::Mat(), hist, 1, histSize, &hranges);
```
# 4. OpenCV 实践应用
### 4.1 图像处理项目
#### 4.1.1 图像去噪和锐化
**图像去噪**
图像去噪是去除图像中不需要的噪声或伪影的过程。OpenCV 提供了多种去噪算法,包括:
- **均值滤波:**通过对图像中的每个像素及其周围像素求平均值来平滑图像。
- **中值滤波:**通过对图像中的每个像素及其周围像素的中值进行替换来去除噪声。
- **高斯滤波:**通过使用高斯核对图像进行卷积来平滑图像,从而模糊噪声。
**代码块:**
```python
import cv2
# 读取图像
image = cv2.imread('noisy_image.jpg')
# 应用均值滤波
denoised_image = cv2.blur(image, (5, 5))
# 显示去噪后的图像
cv2.imshow('Denoised Image', denoised_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.blur()` 函数使用指定的内核大小(5x5)对图像进行均值滤波。
* 滤波后的图像存储在 `denoised_image` 变量中。
* `cv2.imshow()` 函数显示去噪后的图像。
* `cv2.waitKey(0)` 等待用户按任意键关闭图像窗口。
* `cv2.destroyAllWindows()` 关闭所有 OpenCV 窗口。
**图像锐化**
图像锐化是增强图像中边缘和细节的过程。OpenCV 提供了以下锐化算法:
- **拉普拉斯算子:**使用拉普拉斯算子对图像进行卷积,以突出边缘。
- **Sobel 算子:**使用 Sobel 算子对图像进行卷积,以检测水平和垂直边缘。
- **Canny 边缘检测:**使用 Canny 边缘检测算法检测图像中的边缘。
**代码块:**
```python
import cv2
# 读取图像
image = cv2.imread('blurred_image.jpg')
# 应用拉普拉斯算子
sharpened_image = cv2.Laplacian(image, cv2.CV_64F)
# 显示锐化后的图像
cv2.imshow('Sharpened Image', sharpened_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.Laplacian()` 函数使用拉普拉斯算子对图像进行卷积,以锐化图像。
* 锐化后的图像存储在 `sharpened_image` 变量中。
* `cv2.imshow()` 函数显示锐化后的图像。
* `cv2.waitKey(0)` 等待用户按任意键关闭图像窗口。
* `cv2.destroyAllWindows()` 关闭所有 OpenCV 窗口。
### 4.1.2 图像拼接和全景图创建
**图像拼接**
图像拼接是将两张或多张图像合并成一张全景图的过程。OpenCV 提供了以下图像拼接算法:
- **特征匹配:**使用 SIFT 或 SURF 等算法在图像之间找到匹配特征。
- **图像配准:**使用 RANSAC 或 Homography 等算法对图像进行配准。
- **图像融合:**使用加权平均或羽化等技术将图像融合在一起。
**代码块:**
```python
import cv2
# 读取图像
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
# 特征匹配
sift = cv2.SIFT_create()
keypoints1, descriptors1 = sift.detectAndCompute(image1, None)
keypoints2, descriptors2 = sift.detectAndCompute(image2, None)
matches = cv2.FlannBasedMatcher().knnMatch(descriptors1, descriptors2, k=2)
# 图像配准
homography, mask = cv2.findHomography(np.array([keypoints1[m.queryIdx].pt for m in matches[0]]),
np.array([keypoints2[m.trainIdx].pt for m in matches[0]]), cv2.RANSAC, 5.0)
# 图像融合
stitched_image = cv2.warpPerspective(image1, homography, (image1.shape[1] + image2.shape[1], image1.shape[0]))
stitched_image[0:image2.shape[0], image1.shape[1]:image1.shape[1] + image2.shape[1]] = image2
# 显示拼接后的图像
cv2.imshow('Stitched Image', stitched_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.SIFT_create()` 创建一个 SIFT 特征检测器。
* `detectAndCompute()` 函数在图像中检测和计算 SIFT 特征。
* `FlannBasedMatcher().knnMatch()` 使用最近邻算法匹配图像中的特征。
* `findHomography()` 函数使用 RANSAC 算法计算图像之间的同态变换矩阵。
* `warpPerspective()` 函数使用同态变换矩阵将图像配准到全景图中。
* `imshow()` 函数显示拼接后的图像。
* `waitKey(0)` 等待用户按任意键关闭图像窗口。
* `destroyAllWindows()` 关闭所有 OpenCV 窗口。
**全景图创建**
全景图创建是将多个图像拼接成 360 度全景图的过程。OpenCV 提供了以下全景图创建算法:
- **球形投影:**将图像投影到一个球形表面上。
- **立方体投影:**将图像投影到一个立方体表面上。
- **圆柱投影:**将图像投影到一个圆柱表面上。
**代码块:**
```python
import cv2
# 读取图像
images = ['image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg']
# 创建球形全景图
stitcher = cv2.Stitcher_create()
status, stitched_image = stitcher.stitch(images)
# 显示全景图
if status == cv2.STITCHER_OK:
cv2.imshow('Panorama', stitched_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
else:
print('Error: Panorama creation failed.')
```
**逻辑分析:**
* `Stitcher_create()` 创建一个全景图拼接器。
* `stitch()` 函数将图像拼接成全景图。
* `status` 变量表示拼接操作的状态。
* `imshow()` 函数显示全景图。
* `waitKey(0)` 等待用户按任意键关闭图像窗口。
* `destroyAllWindows()` 关闭所有 OpenCV 窗口。
# 5.1 图像处理算法优化
### 5.1.1 多线程处理和 GPU 加速
**多线程处理**
多线程处理可以将图像处理任务分解为多个较小的任务,并同时在多个处理器内核上执行。这可以显著提高图像处理速度,尤其是在处理大型图像或复杂算法时。
**代码块:**
```cpp
#include <opencv2/opencv.hpp>
#include <thread>
#include <vector>
void processImage(cv::Mat& image) {
// 图像处理代码
}
int main() {
cv::Mat image = cv::imread("image.jpg");
// 创建线程池
std::vector<std::thread> threads;
// 将图像分成 4 个部分
std::vector<cv::Mat> parts;
cv::split(image, parts);
// 为每个部分创建线程
for (auto& part : parts) {
threads.emplace_back(std::thread(processImage, std::ref(part)));
}
// 等待所有线程完成
for (auto& thread : threads) {
thread.join();
}
// 合并处理后的部分
cv::merge(parts, image);
// 保存处理后的图像
cv::imwrite("processed_image.jpg", image);
return 0;
}
```
**GPU 加速**
GPU(图形处理单元)专为处理大量并行计算而设计,非常适合图像处理任务。使用 GPU 加速可以进一步提高图像处理速度,尤其是对于需要大量矩阵运算的算法。
**代码块:**
```cpp
#include <opencv2/opencv.hpp>
#include <cuda.h>
void processImageGPU(cv::Mat& image) {
// 将图像上传到 GPU
cudaMemcpy(cudaDevice, image.data, image.total() * image.elemSize(), cudaMemcpyHostToDevice);
// 在 GPU 上执行图像处理代码
// 将处理后的图像从 GPU 下载
cudaMemcpy(image.data, cudaDevice, image.total() * image.elemSize(), cudaMemcpyDeviceToHost);
}
int main() {
cv::Mat image = cv::imread("image.jpg");
// 处理图像
processImageGPU(image);
// 保存处理后的图像
cv::imwrite("processed_image.jpg", image);
return 0;
}
```
### 5.1.2 算法选择和性能评估
**算法选择**
不同的图像处理算法具有不同的优点和缺点。选择合适的算法对于优化性能至关重要。考虑以下因素:
- **算法复杂度:**算法所需的时间和空间复杂度。
- **图像类型:**算法适用于特定类型的图像(例如,灰度图像、彩色图像)。
- **处理目标:**算法旨在解决特定图像处理问题(例如,去噪、边缘检测)。
**性能评估**
性能评估对于确定图像处理算法的效率至关重要。使用以下指标评估性能:
- **执行时间:**算法执行所需的时间。
- **内存使用:**算法所需的内存量。
- **图像质量:**处理后的图像质量与原始图像相比。
**代码块:**
```cpp
#include <opencv2/opencv.hpp>
#include <chrono>
int main() {
cv::Mat image = cv::imread("image.jpg");
// 计时算法执行时间
auto start = std::chrono::high_resolution_clock::now();
cv::GaussianBlur(image, image, cv::Size(5, 5), 0);
auto end = std::chrono::high_resolution_clock::now();
// 计算执行时间
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
// 打印执行时间
std::cout << "执行时间:" << duration.count() << "毫秒" << std::endl;
return 0;
}
```
0
0
相关推荐
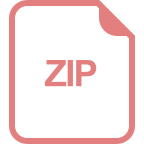
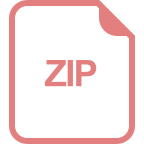
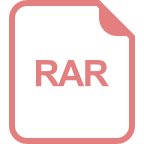





