用c++实现用opencv获取轮廓之后填充轮廓
时间: 2023-05-23 13:07:01 浏览: 96
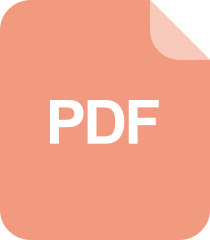
opencv 图像轮廓的实现示例
A:以下是用C语言和OpenCV库实现获取轮廓并填充轮廓的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <opencv2/opencv.hpp>
int main()
{
// 读取图像
cv::Mat img = cv::imread("example.jpg");
if (img.empty()) {
printf("Failed to read image!\n");
return EXIT_FAILURE;
}
// 转为灰度图像
cv::Mat gray;
cv::cvtColor(img, gray, cv::COLOR_BGR2GRAY);
// 获取轮廓
std::vector<std::vector<cv::Point> > contours;
std::vector<cv::Vec4i> hierarchy;
cv::findContours(gray, contours, hierarchy, cv::RETR_TREE, cv::CHAIN_APPROX_SIMPLE);
// 填充轮廓
cv::Scalar color(255, 255, 255);
cv::Mat filled = cv::Mat::zeros(img.size(), img.type());
for (size_t i = 0; i < contours.size(); i++) {
cv::drawContours(filled, contours, i, color, -1, cv::LINE_AA);
}
// 显示结果
cv::imshow("Original", img);
cv::imshow("Filled Contours", filled);
cv::waitKey(0);
return 0;
}
```
在此示例中,我们使用 `cv::imread()` 函数加载要处理的图像。然后,我们将图像转换为灰度图像,以便更容易地获取轮廓。接下来,我们使用 `cv::findContours()` 函数获取图像中的所有轮廓。最后,我们使用 `cv::drawContours()` 函数在新图像上绘制填充轮廓。最终结果将在两个窗口中显示:原始图像以及填充轮廓的新图像。
阅读全文
相关推荐




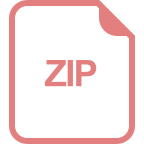
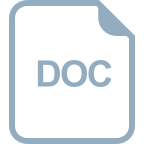
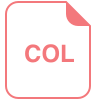
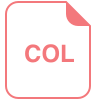


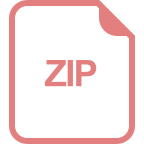
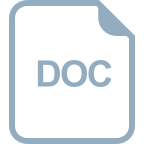
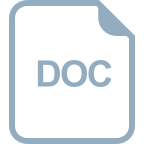


