3. C语言设计实现24秒计时器。要求: (1)倒计时功能 (2)24秒复位键功能 (3)启动/暂停键功能
时间: 2023-12-14 10:35:41 浏览: 44
下面是一个基本的C语言实现,使用了计时器、延时和键盘输入等基本的函数库:
```c
#include <stdio.h>
#include <conio.h>
#include <dos.h>
void countdown(void); // 倒计时
void reset(void); // 复位
void pause(void); // 暂停/启动
int main() {
char ch;
while (1) {
printf("\nPress 'c' to start countdown, 'r' to reset, 'p' to pause.\n");
ch = getch();
switch (ch) {
case 'c':
countdown();
break;
case 'r':
reset();
break;
case 'p':
pause();
break;
default:
printf("Invalid input, try again.\n");
}
}
return 0;
}
void countdown() {
int sec = 24;
while (sec >= 0) {
printf("%d\n", sec);
delay(1000);
sec--;
if (kbhit()) { // 检查是否有键盘输入
if (getch() == 'p') { // 如果是p键,则暂停计时器
printf("Countdown paused.\n");
while (getch() != 'p'); // 等待用户按p键继续
printf("Countdown resumed.\n");
}
}
}
printf("Time's up!\n");
}
void reset() {
printf("24-second timer reset.\n");
}
void pause() {
printf("24-second timer paused.\n");
while (getch() != 'p'); // 等待用户按p键继续
printf("24-second timer resumed.\n");
}
```
其中,`countdown()`函数实现了倒计时功能,每秒输出剩余时间,并通过`kbhit()`函数检查是否有键盘输入(如p键),以实现暂停/启动功能。`reset()`函数实现了复位功能,`pause()`函数实现了暂停/启动功能。
相关推荐
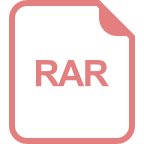
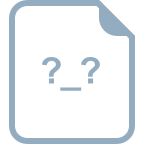
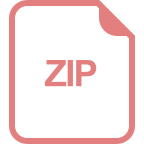











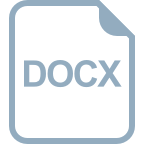
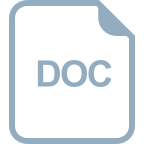
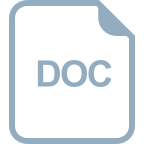