In the context of loops, what is the difference between the "continue" statement and the "break" statement?
时间: 2024-03-10 21:44:44 浏览: 98
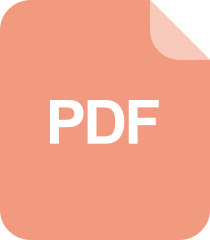
The C Programming Language.pdf

In the context of loops, both "continue" and "break" statements are used to control the flow of the loop, but they have different effects:
- The "continue" statement is used to skip the current iteration of a loop and move on to the next one. When the "continue" statement is encountered in a loop, the remaining code in the loop body is skipped for the current iteration, and the loop moves on to the next iteration. The loop continues to execute until all iterations are completed.
For example, let's say we have a loop that iterates over a list of numbers and we want to skip any negative numbers. We could use the "continue" statement to skip over any negative numbers and move on to the next iteration:
```
numbers = [1, -2, 3, -4, 5]
for num in numbers:
if num < 0:
continue
print(num)
```
Output:
```
1
3
5
```
- The "break" statement is used to terminate a loop early. When the "break" statement is encountered in a loop, the loop terminates immediately, and the program execution moves on to the next statement after the loop.
For example, let's say we have a loop that iterates over a list of numbers and we want to stop the loop as soon as we find a negative number. We could use the "break" statement to terminate the loop early:
```
numbers = [1, -2, 3, -4, 5]
for num in numbers:
if num < 0:
break
print(num)
```
Output:
```
1
```
In summary, the "continue" statement skips the current iteration of a loop and moves on to the next one, while the "break" statement terminates the loop early and moves on to the next statement after the loop.
阅读全文
相关推荐
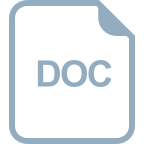
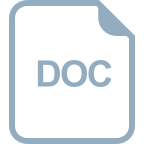
















