Using the fact that for_each returns the unary function passed as a parameter, write a function MyAccumulate that accepts as parameters a range of vector<int>::iterators and an initial value, then returns the sum of all of the values in the range, starting at the specified value. Do not use any loops – instead, use for_each and a custom functor class that performs the addition.
时间: 2024-02-19 15:03:46 浏览: 99
Sure, here is the implementation of the `MyAccumulate` function:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
class AddFunctor {
public:
AddFunctor(int val) : sum(val) {}
void operator()(int val) {
sum += val;
}
int getResult() const { return sum; }
private:
int sum;
};
int MyAccumulate(std::vector<int>::iterator begin, std::vector<int>::iterator end, int initVal) {
AddFunctor addFunc(initVal);
std::for_each(begin, end, addFunc);
return addFunc.getResult();
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int sum = MyAccumulate(vec.begin(), vec.end(), 10);
std::cout << "Sum: " << sum << std::endl; // Output: Sum: 25
return 0;
}
```
The `AddFunctor` class is a custom functor that performs the addition. It has an internal variable `sum` that holds the current sum, and the `operator()` method that updates the sum by adding the input argument. The `getResult` method returns the final sum.
The `MyAccumulate` function takes the range of vector iterators and the initial value as input parameters. It creates an instance of the `AddFunctor` class with the initial value, then applies the functor to each element in the range using `std::for_each`. Finally, it returns the result by calling the `getResult` method of the functor.
阅读全文
相关推荐
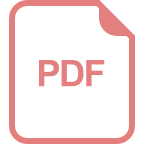
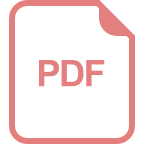















