There are n creatures on the plane, creature number i is a point with coordinates (xi,yi). Your goal is to establish a protective field in a shape of a circle with the minimal possible radius that will cover at least half of those creatures, i.e. ⌈n2⌉. Given that you can choose any point (possibly with non-integers coordinates) as a center of the field, determine the optimal position and radius of the field. Input The first line of the input contains an integer n — the number of creatures (1≤n≤400). The next n lines contain n pairs of real numbers xi and yi — the coordinates of each creature (−109≤xi,yi≤109). The creatures are lazy and do not move, so their positions are constant. Output Output three numbers — the center of the protective field of the minimum radius that covers at least half of the creatures, and the radius itself. All numbers should be printed with an absolute or relative error not exceeding 10−6. Examples inputCopy 8 4 3 3 4 -3 4 -4 3 -4 -3 -3 -4 3 -4 4 -3 outputCopy 0 3 4 inputCopy 4 1.5 1.5 1.5 -1.5 -1.5 -1.5 -1.5 1.5 outputCopy 1.5 0 1.5
时间: 2024-04-17 14:25:13 浏览: 93
To solve this problem, we can iterate over all possible center points of the protective field and calculate the minimum radius required to cover at least half of the creatures.
Here's a step-by-step approach to solve the problem:
1. Read the input values: the number of creatures, n, and the coordinates of each creature.
2. Initialize variables to store the optimal position and radius of the field. Let's call them `optimal_x`, `optimal_y`, and `optimal_radius`. Set `optimal_radius` to a large value initially.
3. Iterate over all possible center points of the field. You can use nested loops to iterate over x and y coordinates within a reasonable range.
4. For each center point, calculate the distance from that point to all creatures. You can use the distance formula: `distance = sqrt((x - xi)^2 + (y - yi)^2)`.
5. Sort the distances in ascending order.
6. Calculate the radius required to cover at least half of the creatures. This can be done by taking the distance at index `ceil(n/2)` - 1, where `ceil()` is the ceiling function.
7. If the calculated radius is smaller than the current `optimal_radius`, update `optimal_x`, `optimal_y`, and `optimal_radius` with the current center point and radius.
8. After iterating over all possible center points, print the values of `optimal_x`, `optimal_y`, and `optimal_radius` as the output.
Here's a Python implementation of the above approach:
```python
import math
n = int(input())
creatures = []
for _ in range(n):
x, y = map(float, input().split())
creatures.append((x, y))
optimal_x = 0
optimal_y = 0
optimal_radius = float('inf')
for x in range(-100, 101):
for y in range(-100, 101):
distances = []
for creature in creatures:
xi, yi = creature
distance = math.sqrt((x - xi)**2 + (y - yi)**2)
distances.append(distance)
distances.sort()
radius = distances[math.ceil(n/2) - 1]
if radius < optimal_radius:
optimal_x = x
optimal_y = y
optimal_radius = radius
print(optimal_x, optimal_y, optimal_radius)
```
This implementation has a time complexity of O(n^3) since it iterates over all possible center points and calculates the distances for each point. However, since the maximum value of n is 400, the solution should run efficiently within the given constraints.
阅读全文
相关推荐
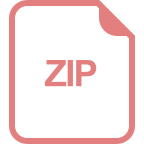
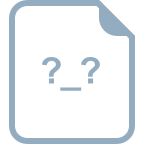
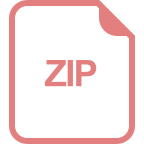
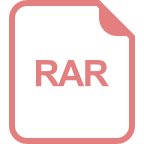
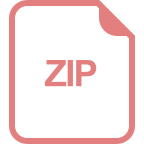
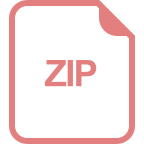
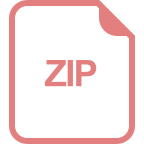
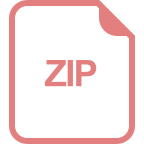
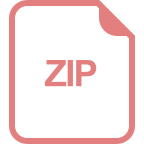
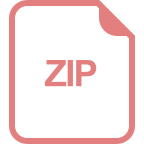




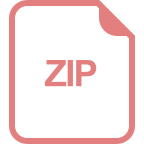