用C++写一个生存游戏
时间: 2023-12-18 12:05:41 浏览: 43
好的,生存游戏可以理解为一种模拟人类在野外生存的游戏,我们可以采用以下的设计思路:
1. 定义生物基类,包括生物的基本属性和行为;
2. 定义人类类,包括人类独特的属性和行为,比如饮食、睡眠、狩猎等;
3. 定义动物类,包括动物的属性和行为,比如食性、繁殖等;
4. 定义地图类,用于表示游戏世界,包括地形、环境、资源等;
5. 定义游戏类,用于控制游戏的整个流程,包括初始化、循环、退出等。
以下是用C++实现的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
// 生物基类
class Creature {
public:
Creature(int health, int age) : health_(health), age_(age) {}
virtual ~Creature() {}
virtual void Eat() = 0; // 吃
virtual void Sleep() = 0; // 睡觉
virtual void Move() = 0; // 移动
virtual void Die() { health_ = 0; } // 死亡
int GetHealth() const { return health_; }
int GetAge() const { return age_; }
protected:
int health_; // 生命值
int age_; // 年龄
};
// 人类类
class Human : public Creature {
public:
Human(int health, int age, int hunger) : Creature(health, age), hunger_(hunger) {}
void Eat() override { hunger_ = 0; health_ += 10; }
void Sleep() override { health_ += 5; }
void Move() override { health_ -= 5; hunger_ += 5; }
int GetHunger() const { return hunger_; }
private:
int hunger_; // 饥饿度
};
// 动物类
class Animal : public Creature {
public:
Animal(int health, int age, int hunger) : Creature(health, age), hunger_(hunger) {}
void Eat() override { hunger_ = 0; health_ += 5; }
void Sleep() override { health_ += 3; }
void Move() override { health_ -= 3; hunger_ += 3; }
int GetHunger() const { return hunger_; }
private:
int hunger_; // 饥饿度
};
// 地图类
class Map {
public:
Map(int width, int height) : width_(width), height_(height) {}
void AddCreature(Creature* creature, int x, int y) {
if (x >= 0 && x < width_ && y >= 0 && y < height_) {
creatures_[x][y].push_back(creature);
}
}
void RemoveCreature(Creature* creature, int x, int y) {
if (x >= 0 && x < width_ && y >= 0 && y < height_) {
auto& creatures = creatures_[x][y];
creatures.erase(std::remove(creatures.begin(), creatures.end(), creature), creatures.end());
}
}
std::vector<Creature*>& GetCreatures(int x, int y) {
return creatures_[x][y];
}
private:
int width_;
int height_;
std::vector<Creature*> creatures_[100][100];
};
// 游戏类
class Game {
public:
Game() : map_(100, 100) {}
void Init() {
srand(static_cast<unsigned int>(time(nullptr)));
// 初始化人类和动物
for (int i = 0; i < 10; ++i) {
int x = rand() % 100;
int y = rand() % 100;
int age = rand() % 100;
int hunger = rand() % 100;
if (i < 5) {
humans_.push_back(new Human(100, age, hunger));
map_.AddCreature(humans_.back(), x, y);
} else {
animals_.push_back(new Animal(50, age, hunger));
map_.AddCreature(animals_.back(), x, y);
}
}
}
void Run() {
// 游戏循环
while (true) {
// 更新人类状态
for (auto& human : humans_) {
human->Eat();
human->Sleep();
human->Move();
if (human->GetHealth() <= 0) {
human->Die();
map_.RemoveCreature(human, 0, 0);
}
}
// 更新动物状态
for (auto& animal : animals_) {
animal->Eat();
animal->Sleep();
animal->Move();
if (animal->GetHealth() <= 0) {
animal->Die();
map_.RemoveCreature(animal, 0, 0);
}
}
// 显示游戏状态
std::system("clear");
for (int y = 0; y < 100; ++y) {
for (int x = 0; x < 100; ++x) {
auto& creatures = map_.GetCreatures(x, y);
if (creatures.empty()) {
std::cout << ".";
} else if (creatures.size() == 1) {
if (dynamic_cast<Human*>(creatures[0])) {
std::cout << "@";
} else {
std::cout << "*";
}
} else {
std::cout << "#";
}
}
std::cout << std::endl;
}
// 暂停一段时间
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
}
private:
std::vector<Human*> humans_;
std::vector<Animal*> animals_;
Map map_;
};
int main() {
Game game;
game.Init();
game.Run();
return 0;
}
```
这是一个简单的生存游戏,其中人类和动物可以在地图上移动、寻找食物、睡觉等,游戏会按照一定的时间间隔不断更新状态,并显示在屏幕上。如果生命值归零,则会死亡。
相关推荐
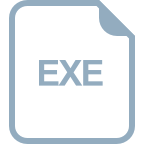
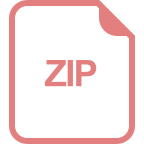
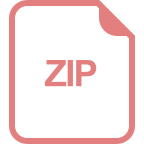














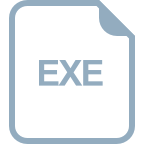