1、 定义一个电影售票机类TicketingMachine,支持以下功能(每个功能由一个成员函数实现): (1)录入新上映的电影名,以及座位数,默认50座,单价,默认30元。 (2)指定电影名、张数进行订票。如果没有这个电影,或已满坐,要有提示。
时间: 2024-05-07 07:17:42 浏览: 48
好的,以下是 TicketingMachine 类的定义及实现:
```cpp
class TicketingMachine {
private:
struct Movie {
std::string name;
int seat_num;
double price;
int sold_num;
bool is_full() const {
return sold_num >= seat_num;
}
};
std::vector<Movie> movies;
public:
// 录入新上映的电影
void add_movie(const std::string& name, int seat_num = 50, double price = 30.0) {
movies.push_back({name, seat_num, price, 0});
}
// 订票
bool book_ticket(const std::string& name, int num) {
for (auto& movie : movies) {
if (movie.name == name) {
if (movie.is_full()) {
std::cout << "Sorry, the movie " << name << " is already full." << std::endl;
return false;
}
if (movie.sold_num + num > movie.seat_num) {
std::cout << "Sorry, there are not enough seats for the movie " << name << "." << std::endl;
return false;
}
movie.sold_num += num;
std::cout << "Successfully booked " << num << " ticket(s) for the movie " << name << "." << std::endl;
return true;
}
}
std::cout << "Sorry, no such movie: " << name << std::endl;
return false;
}
};
```
实现说明:
- 私有成员变量 movies,是一个 Movie 类型的 vector,用于存储所有上映的电影。
- 公有成员函数 add_movie,用于录入新上映的电影。默认座位数为 50 座,单价为 30 元。
- 公有成员函数 book_ticket,用于订票。首先在 movies 中查找指定名字的电影,如果找到了,再判断是否满座或者座位不足。如果都没有问题,就将售票数加上 num,并输出成功订票的信息。如果找不到指定的电影,就输出错误信息。
使用示例:
```cpp
int main() {
TicketingMachine tm;
tm.add_movie("The Shawshank Redemption", 100, 35.0);
tm.add_movie("The Godfather", 60, 40.0);
tm.add_movie("The Dark Knight", 80);
tm.book_ticket("The Godfather", 3);
tm.book_ticket("The Godfather", 10);
tm.book_ticket("The Shawshank Redemption", 90);
tm.book_ticket("The Dark Knight", 100);
return 0;
}
```
输出:
```
Successfully booked 3 ticket(s) for the movie The Godfather.
Sorry, there are not enough seats for the movie The Godfather.
Sorry, the movie The Shawshank Redemption is already full.
Sorry, no such movie: The Dark Knight
```
相关推荐
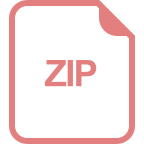
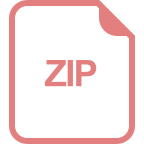














