pygame中怎么将img图片设置为rect形式
时间: 2023-04-02 14:03:10 浏览: 184
你可以使用pygame中的函数pygame.image.load()来加载图片,然后使用pygame.Rect()函数将图片转换为矩形形式。具体代码如下:
```
import pygame
pygame.init()
# 加载图片
img = pygame.image.load("image.png")
# 将图片转换为矩形形式
rect = img.get_rect()
# 显示图片
screen = pygame.display.set_mode((800, 600))
screen.blit(img, rect)
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
```
这样就可以将图片设置为矩形形式并显示在屏幕上了。
相关问题
pygame缩放图片
可以使用pygame中的transform模块来缩放图片。具体步骤如下:
1.导入pygame和sys模块
```python
import pygame
import sys
```
2.初始化pygame
```python
pygame.init()
```
3.设置窗口大小
```python
WIDTH, HEIGHT = 640, 480
```
4.创建窗口
```python
screen = pygame.display.set_mode((WIDTH, HEIGHT))
```
5.加载图片
```python
image = pygame.image.load('in.jpg')
```
6.缩放图片
```python
scale_img = pygame.transform.scale(image, (100, 100))
```
7.获取缩放后图片的位置和大小
```python
img_rect = scale_img.get_rect()
```
8.将缩放后图片的中心移动到窗口中心
```python
img_rect.center = (WIDTH//2, HEIGHT//2)
```
9.将缩放后的图片绘制到窗口中
```python
screen.blit(scale_img, img_rect)
```
10.刷新窗口
```python
pygame.display.flip()
```
完整代码如下:
```python
import pygame
import sys
pygame.init()
WIDTH, HEIGHT = 640, 480
screen = pygame.display.set_mode((WIDTH, HEIGHT))
image = pygame.image.load('in.jpg')
scale_img = pygame.transform.scale(image, (100, 100))
img_rect = scale_img.get_rect()
img_rect.center = (WIDTH//2, HEIGHT//2)
screen.blit(scale_img, img_rect)
pygame.display.flip()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
```
pygame使用Sprite实现图片动画
使用`pygame.sprite.Sprite`类可以更方便地管理和绘制多个图片对象。下面是一个使用Sprite类实现图片动画的示例代码:
```python
import pygame
pygame.init()
# 窗口大小
win_width = 500
win_height = 500
# 创建窗口
win = pygame.display.set_mode((win_width, win_height))
pygame.display.set_caption("Sprite Animation")
# 加载图片
img1 = pygame.image.load("img1.png")
img2 = pygame.image.load("img2.png")
img3 = pygame.image.load("img3.png")
# 设置动画帧数
fps = 10
# 创建时钟
clock = pygame.time.Clock()
# 创建精灵组
sprites = pygame.sprite.Group()
# 定义精灵类
class MySprite(pygame.sprite.Sprite):
def __init__(self, img, x, y):
super().__init__()
self.image = img
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
# 创建精灵对象
sprite1 = MySprite(img1, 50, 50)
sprite2 = MySprite(img2, 150, 50)
sprite3 = MySprite(img3, 250, 50)
sprites.add(sprite1, sprite2, sprite3)
# 运行游戏
run = True
while run:
# 控制帧数
clock.tick(fps)
# 监听事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
# 重绘窗口
win.fill((255, 255, 255))
sprites.draw(win)
pygame.display.update()
# 退出pygame
pygame.quit()
```
在上述代码中,我们首先加载了三张图片,并设置了动画帧数。然后创建了一个精灵组,并定义了一个精灵类,该类继承自`pygame.sprite.Sprite`类,并重写了`__init__`方法,用于初始化精灵对象的属性。然后创建了三个精灵对象,将它们添加到精灵组中。在每一帧重新绘制窗口时,我们只需要调用`sprites.draw(win)`方法即可将精灵组中的所有精灵对象绘制到窗口上。
使用Sprite类实现图片动画时,我们可以更方便地管理和操作多个图片对象,而且代码也更加简洁和易读。
相关推荐
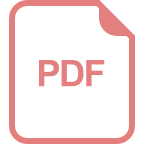
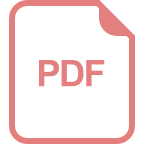












